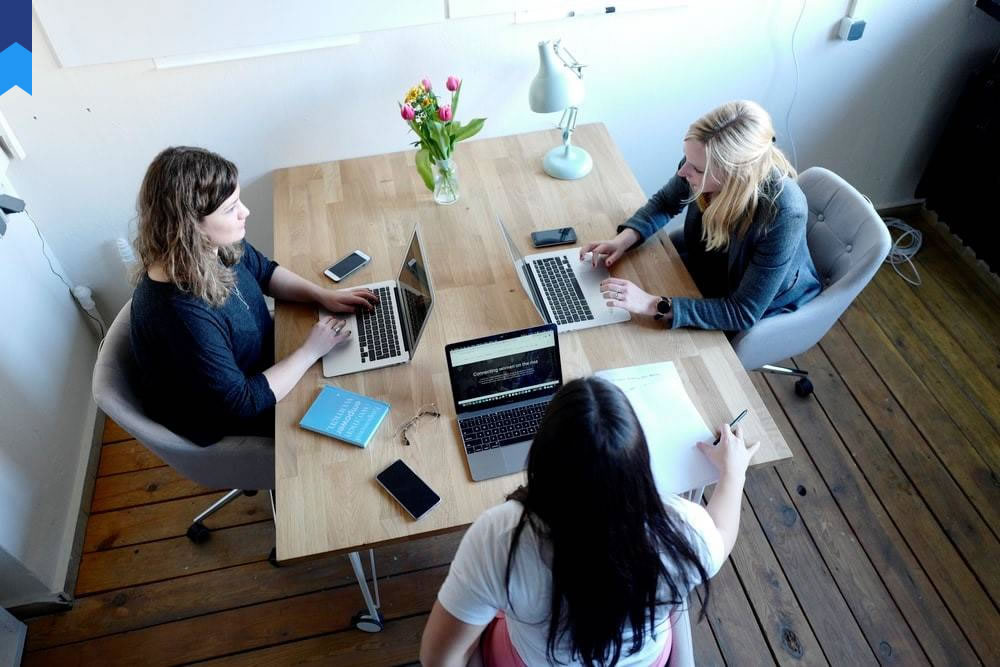
Transform Your Code Through Finite Automata: A Practical Guide
Automata theory, often perceived as a purely theoretical subject, holds the key to unlocking elegant and efficient solutions in various coding challenges. This practical guide delves into the transformative power of finite automata, moving beyond the basic overviews and exploring their innovative applications in modern software development.
Understanding the Fundamentals of Finite Automata
Finite automata (FA) are mathematical models used to represent computational systems with finite memory. They consist of states, transitions between states based on input symbols, and an acceptance criterion. A crucial concept is the distinction between deterministic finite automata (DFA) and non-deterministic finite automata (NFA). DFAs have a unique next state for every input symbol in each state, while NFAs allow for multiple transitions from a single state on the same input symbol. The power of NFAs lies in their ability to represent complex patterns more concisely, often leading to simpler designs. Consider the task of validating email addresses. A DFA would require a large number of states to handle all possible variations, while an NFA can achieve the same result with fewer states by utilizing non-determinism.
Case Study 1: Lexical analysis in compilers utilizes DFAs extensively to identify tokens in the source code. Each state represents a stage of token recognition, with transitions based on the input characters. This approach ensures efficient and accurate parsing of the code. Case Study 2: Network packet filtering relies heavily on DFAs. The router examines the packet headers and traverses states based on the information found, accepting or rejecting the packet based on predefined rules. This is crucial for network security and performance. The choice between DFA and NFA depends on the specific application requirements. For simple pattern matching, DFAs might suffice; for complex patterns or when minimizing the number of states is a priority, NFAs, which can be converted to equivalent DFAs using the subset construction algorithm, become more advantageous.
Regular expressions, a powerful tool for pattern matching, are closely related to finite automata. Every regular expression can be converted into an equivalent NFA, and vice versa. This equivalence allows developers to leverage the strengths of both formalisms. The succinctness of regular expressions makes them ideal for specifying patterns, while the computational model of finite automata provides a clear path for efficient implementation. For example, many programming languages use regular expressions for input validation, search and replace operations, and text processing tasks. Libraries like PCRE (Perl Compatible Regular Expressions) are widely used and demonstrate the practical power of this connection.
The efficiency of DFA-based algorithms stems from their deterministic nature. Given an input and a current state, the next state is uniquely defined. This eliminates the need for backtracking or exploration of multiple paths, resulting in linear-time complexity. This efficiency is paramount in applications where performance is critical, such as network packet processing and real-time systems. Regular languages, the set of languages recognized by FAs, constitute a foundational element of theoretical computer science. They provide a framework for understanding and classifying the complexity of computational problems.
Implementing Finite Automata in Practice
Transition tables are the most common way to represent finite automata in code. A two-dimensional array, where rows represent states and columns represent input symbols, stores the next-state information. The implementation can be easily extended to include output actions associated with transitions, leading to the concept of a Mealy or Moore machine. In a Mealy machine, the output depends on both the current state and the input symbol, while in a Moore machine, the output depends solely on the current state. This flexibility allows the design of finite-state machines for a wide range of applications requiring different output mechanisms.
Case Study 1: Consider a simple vending machine. A Moore machine can model this system effectively. The states represent the machine's internal state (e.g., awaiting payment, dispensing product), while the outputs represent actions like accepting money or dispensing goods. The machine transitions between states based on input events like coin insertion or button presses. The output is determined solely by the current state. Case Study 2: A lexical analyzer in a compiler can be designed as a Mealy machine. The transitions depend on input characters, and the output is generated based on the current state and the input character (e.g., identifying an identifier, a keyword, or an operator).
Programming languages offer various ways to implement finite automata. State machines can be explicitly coded using conditional statements, or more sophisticated approaches can leverage data structures like dictionaries or maps to represent transition tables. Object-oriented programming (OOP) offers an elegant way to structure state machines by representing each state as a class and transitions as method calls. OOP simplifies the development and management of complex state machines, particularly in large software systems.
State machine frameworks and libraries further enhance the ease of developing and deploying finite-state machines. They provide pre-built functionalities for state management, transition handling, and event processing. This simplifies the development process and allows developers to focus on the application logic rather than the low-level details of state machine implementation. Many languages have libraries specifically designed to aid in the implementation of state machines, promoting code reusability and maintainability.
Careful design and implementation are key to developing efficient and reliable finite automata-based systems. The choice of data structures, the organization of the state machine code, and error handling strategies greatly influence the performance and robustness of the system. Well-structured code and thorough testing are crucial to ensure that the state machine operates correctly in all scenarios.
Advanced Techniques and Applications
Beyond basic pattern matching, finite automata find applications in diverse fields. In natural language processing (NLP), hidden Markov models (HMMs), a type of probabilistic finite automaton, are used for tasks like part-of-speech tagging and speech recognition. HMMs incorporate probabilistic elements to handle uncertainty and noise in the input data. This statistical approach makes them well-suited for real-world applications where perfect data is unavailable.
Case Study 1: Google's speech recognition system utilizes HMMs to model the probabilistic transitions between phonemes in speech. The system considers multiple possible pronunciations and selects the most likely sequence based on the input audio. Case Study 2: Part-of-speech tagging algorithms often employ HMMs to determine the grammatical role of each word in a sentence. The HMM learns the probabilities of different word sequences and assigns the most probable tags to each word. This probabilistic nature allows for robustness against noisy or ambiguous input data.
In bioinformatics, finite automata are used for sequence analysis, pattern detection in DNA or protein sequences, and gene prediction. The regular expression-based approach is particularly well-suited for identifying specific patterns, motifs, or conserved regions within biological sequences. These techniques are essential for genomic research and drug discovery.
Case Study 1: Identifying specific DNA sequences responsible for certain genetic disorders. Case Study 2: Detecting conserved protein domains that indicate functional similarities between proteins.
Furthermore, the concepts of finite automata extend to more complex models, such as pushdown automata and Turing machines. Pushdown automata add a stack to the finite automaton, allowing them to recognize context-free languages. Turing machines, the most powerful model of computation, form the foundation of theoretical computer science and provide a framework for understanding the limits of computation. These advanced models extend the capabilities of finite automata to solve more complex problems that lie beyond the reach of regular languages.
The use of these advanced models, however, often introduces additional complexity and computational cost. For many applications, the power and efficiency of finite automata remain sufficient, making them a valuable tool for software developers across various domains.
Optimization and Efficiency Considerations
Minimizing the number of states in a finite automaton is crucial for efficiency, especially in resource-constrained environments. Algorithms like the Hopcroft minimization algorithm can reduce the number of states in a DFA while preserving its functionality. This reduction improves both space and time complexity, leading to faster and more memory-efficient implementations. Minimization algorithms ensure that the resulting DFA is the smallest possible equivalent automaton, optimizing resource usage.
Case Study 1: Network routers employing DFA-based packet filtering benefit significantly from state minimization. A reduced number of states translates to faster processing and less memory consumption. This is particularly crucial for high-traffic networks. Case Study 2: Lexical analyzers in compilers can benefit from state minimization as it can significantly reduce the time and space complexity of the tokenization process.
Careful selection of data structures also plays a significant role in the efficiency of finite automata implementations. Using appropriate data structures like hash tables or tries can accelerate state transitions and input symbol lookup. This efficient data access improves the performance of the automaton, particularly in scenarios with large state spaces and diverse input alphabets. Efficient data structures improve the overall speed and responsiveness of the system.
Case Study 1: Using hash tables for transition table lookup greatly reduces the time required to determine the next state during transitions, especially in large state machines. Case Study 2: Trie-based structures are well-suited for efficiently searching and processing strings, making them suitable for applications like text processing and pattern matching.
Parallel processing techniques can further enhance the performance of finite automata, particularly in applications with high throughput requirements. By distributing the processing of input data across multiple processors, the overall processing time can be significantly reduced. This parallelism is especially beneficial for handling massive input streams efficiently.
Case Study 1: In network security, parallel processing can be used to accelerate the inspection of network packets. Case Study 2: In bioinformatics, parallel processing allows for faster analysis of large genomic datasets.
Future Trends and Emerging Applications
The use of finite automata is expanding into new areas, driven by advancements in computing power and the emergence of new applications. In the Internet of Things (IoT), finite automata are used to model the behavior of smart devices, manage resource allocation, and coordinate communication among interconnected devices. The deterministic nature of finite automata is well-suited for managing real-time interactions and controlling resource usage in constrained environments.
Case Study 1: Finite automata are used to model the state transitions in smart home devices like thermostats and lighting systems. Case Study 2: In industrial automation, finite automata are employed to control manufacturing processes and coordinate the actions of robotic systems.
Machine learning is also influencing the development of more sophisticated finite automata. Techniques like reinforcement learning can be used to train finite automata to learn optimal behaviors and adapt to changing environments. This adaptability allows the creation of self-learning automata that can improve their performance over time based on experience. This integration of machine learning provides powerful methods to create dynamic and adaptive systems.
Case Study 1: Reinforcement learning can be used to train finite automata to optimize resource allocation in a network. Case Study 2: In robotics, reinforcement learning can be used to train finite automata to control robots and adapt to unpredictable environments.
The combination of finite automata with other computational models, such as neural networks, is opening new avenues for creating hybrid systems that leverage the strengths of both approaches. This synergy allows for the development of more powerful and flexible systems that can handle complex tasks that lie beyond the capabilities of individual models. These hybrid models combine the precision of automata with the learning capabilities of neural networks.
Case Study 1: Hybrid systems combining finite automata and neural networks are used for natural language processing. Case Study 2: Hybrid systems are used in autonomous driving to integrate sensor data and control vehicle actions.
Conclusion
Finite automata, often underestimated, represent a powerful and versatile tool in the software developer's arsenal. This exploration of their practical applications, from fundamental concepts to advanced techniques, showcases their importance in various fields. Understanding and leveraging finite automata is not just about theoretical knowledge but about creating efficient, robust, and scalable software solutions. By mastering the techniques described, developers can significantly enhance the design and implementation of their projects across diverse application domains, from compilers and network systems to artificial intelligence and beyond.
The future of finite automata is bright, marked by continued integration with machine learning and other advanced computational models. As computing power continues to increase and new applications emerge, the versatility and power of finite automata will undoubtedly remain relevant and indispensable in the ever-evolving landscape of software development. This warrants continued exploration of their capabilities and the potential for innovative applications.
The ability to design, implement, and optimize finite automata is a valuable skill for any software developer seeking to create elegant and efficient solutions to complex problems. By mastering these techniques, developers can unlock the full potential of finite automata and transform their code into robust and scalable systems.