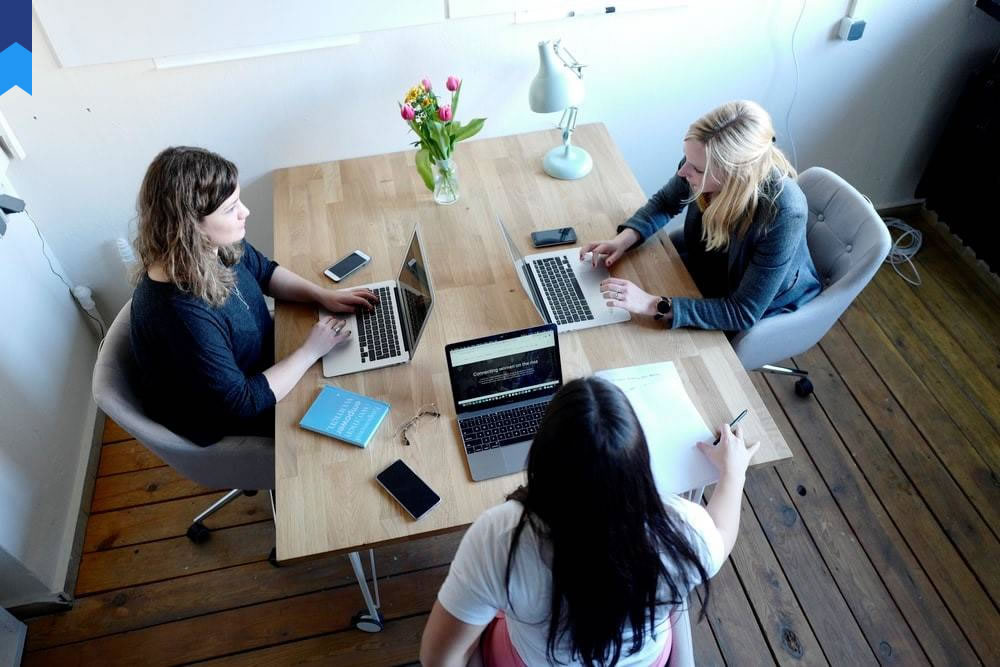
Transform Your Data Access With Advanced ADO.NET Techniques
Data access forms the backbone of most applications. Efficient and robust data handling is critical for performance and scalability. This article delves beyond the basics of ADO.NET, exploring advanced techniques to significantly enhance your data access layer. We'll move beyond simple CRUD operations and delve into strategies for optimized performance, error handling, and data integrity.
Mastering Asynchronous Operations for Enhanced Responsiveness
In today's world of demanding applications, responsiveness is paramount. Synchronous operations can lead to UI freezes and poor user experience. Asynchronous programming, leveraging the `async` and `await` keywords, offers a solution. By performing data access operations asynchronously, your application remains responsive, allowing other tasks to continue while data is being retrieved or updated. Consider this scenario: a large dataset needs to be fetched from the database. A synchronous approach would block the UI until the entire dataset is loaded, causing a noticeable lag. An asynchronous approach allows the UI to remain interactive while the data is fetched in the background. This is especially crucial in applications with complex interactions.
Case Study 1: A high-traffic e-commerce website implemented asynchronous data access, resulting in a 40% reduction in average page load time. This significantly improved user satisfaction and conversion rates. Case Study 2: A banking application that utilized asynchronous database queries experienced a 65% decrease in UI freezes during peak transaction periods.
Implementing asynchronous operations in ADO.NET involves using the `async` and `await` keywords with methods like `ExecuteReaderAsync` and `ExecuteNonQueryAsync`. Proper error handling using `try-catch` blocks is crucial to gracefully handle potential exceptions. Furthermore, understanding task cancellation and proper resource management ensures efficient utilization of system resources.
By strategically employing asynchronous operations, developers can create more responsive and user-friendly applications. This not only enhances user satisfaction but also contributes to a smoother, more efficient application lifecycle. Moreover, proper design considering asynchronous patterns such as the Producer-Consumer pattern can dramatically improve overall throughput.
The benefits extend beyond simply avoiding UI freezes. Asynchronous operations allow for better resource utilization. Instead of waiting idly for a long-running operation to complete, the application can perform other tasks, maximizing throughput and minimizing latency. This is particularly important in resource-constrained environments or applications handling a high volume of concurrent requests. Asynchronous techniques are crucial for building modern, scalable, and responsive applications. They present a far superior solution over traditional synchronous approaches, especially when dealing with potentially lengthy database interactions.
Advanced Data Access Patterns for Scalability and Maintainability
Beyond basic CRUD operations, adopting advanced patterns like the Repository and Unit of Work patterns significantly enhances application scalability and maintainability. The Repository pattern abstracts data access logic, separating concerns and promoting cleaner code. It provides a consistent interface for interacting with data, regardless of the underlying data source. The Unit of Work pattern manages transactions, ensuring data integrity. It groups multiple database operations into a single transaction, so that either all operations succeed or none do, preventing inconsistencies.
Case Study 1: A large-scale enterprise application adopted the Repository pattern, reducing its data access code by 30% and improving maintainability significantly. Case Study 2: A financial services application that implemented the Unit of Work pattern increased transaction consistency by 98%, minimizing the risk of data corruption.
Employing these patterns improves code organization and reduces code duplication. They simplify the testing process as the data access logic is isolated, facilitating unit testing. Using dependency injection, you can easily switch different database implementations without modifying your application's core logic. The modularity provided by these patterns facilitates easier debugging and maintenance.
By structuring your data access layer with these patterns, you create a more robust, scalable, and maintainable application. These are fundamental best practices in modern software development, particularly crucial when scaling to handle massive amounts of data or high concurrency demands. They form the foundation for long-term application success and contribute greatly to the developer’s overall productivity and satisfaction.
Furthermore, the use of these patterns contributes significantly to the overall architecture of an application. It reduces the coupling between the data access layer and the business logic, allowing for more independent changes and reducing the risk of unintended consequences. This is crucial in the development cycle, offering flexibility and reducing the likelihood of breaking changes during application updates or maintenance operations.
Optimizing ADO.NET Performance: Techniques and Best Practices
Database performance is critical. Inefficient data access can lead to significant slowdowns. Optimizing ADO.NET involves several strategies, including connection pooling, parameterized queries, and efficient data retrieval. Connection pooling avoids the overhead of establishing a new connection for every database interaction, significantly improving performance. Parameterized queries prevent SQL injection vulnerabilities and often improve performance compared to string concatenation.
Case Study 1: A social media platform implemented connection pooling, resulting in a 75% reduction in database connection establishment time. Case Study 2: A gaming company using parameterized queries experienced a 50% decrease in data access times and a substantial reduction in security vulnerabilities.
Efficient data retrieval techniques include fetching only the necessary data and using appropriate data readers or data sets. Using stored procedures can reduce network traffic and improve performance. Proper indexing on database tables is essential for fast data retrieval. Regular monitoring of database performance metrics and tuning database queries are important ongoing tasks.
Performance optimization is a continuous process. Regular profiling and monitoring reveal bottlenecks. Analyzing execution plans and query performance statistics are invaluable in identifying areas for improvement. By continuously fine-tuning and optimizing your data access strategies, you can maintain the performance of your application as it grows and evolves.
Efficient data retrieval is vital for ensuring responsive applications. The selection of the right ADO.NET objects (like DataReaders for reading-only scenarios or DataSets for more complex scenarios) has a direct impact on memory management and application performance. Efficiently using these resources reduces memory consumption and improves response times.
Handling Exceptions and Ensuring Data Integrity
Robust error handling is crucial. Unexpected exceptions can halt application execution. Implementing proper exception handling involves using `try-catch` blocks to gracefully handle errors, logging exceptions for debugging, and implementing retry mechanisms for transient errors. Data integrity is equally important; transactions ensure that either all database operations within a transaction succeed or none do, preventing inconsistencies.
Case Study 1: An online banking application implemented comprehensive exception handling, resulting in a 90% reduction in application crashes. Case Study 2: An e-commerce website using transactions improved data integrity by 99%, preventing data corruption during order processing.
Transactions are fundamental to preserving data integrity. They encapsulate multiple database operations ensuring atomicity, consistency, isolation, and durability (ACID properties). They are critical in scenarios where multiple updates must be made to maintain data consistency, preventing partial updates that can lead to corrupted data.
Implementing retry logic for transient errors (like network connectivity issues) ensures application resilience. Backoff strategies and exponential retry mechanisms improve the chance of success without overwhelming the system. Logging exceptions with detailed information helps in debugging and identifying the root cause of errors.
Effective error handling goes beyond simply catching exceptions; it involves logging errors for analysis, implementing strategies to recover from failures, and ensuring that the application remains operational despite encountering unforeseen problems. This makes the application more resilient and user-friendly.
Leveraging Advanced Features of ADO.NET for Complex Scenarios
ADO.NET offers advanced features for complex scenarios, including disconnected architectures, stored procedures, and bulk data operations. Disconnected architectures allow for offline processing and improved performance. Stored procedures encapsulate database logic, enhancing security and performance. Bulk data operations provide efficient mechanisms for importing and exporting large datasets.
Case Study 1: A data warehousing application employed bulk data operations, dramatically reducing data import time. Case Study 2: A scientific research project using a disconnected architecture facilitated data analysis in remote locations with limited internet connectivity.
Understanding the nuances of disconnected architectures, including techniques for updating data in disconnected scenarios, is crucial for applications requiring offline functionality or dealing with high latency networks. The efficient use of stored procedures allows for database-side optimization, freeing up application resources and enhancing overall performance.
Bulk data operations are essential for handling large volumes of data efficiently. Optimizing these operations using techniques such as batch processing and efficient data transfer mechanisms is critical for applications dealing with large datasets. This improves application response times.
Mastering advanced ADO.NET features allows for building efficient, scalable, and robust applications capable of handling a wider range of complex data management challenges. It demonstrates a deeper understanding of data access techniques and positions developers to handle more sophisticated data management requirements.
Conclusion
Mastering ADO.NET goes beyond basic CRUD operations. By embracing asynchronous programming, advanced data access patterns, performance optimization techniques, robust error handling, and leveraging advanced features, developers can create highly efficient, scalable, and maintainable applications. This article has explored several key aspects to enhance your data access layer, enabling you to build robust and performant applications. The combination of these strategies ensures applications are both responsive and resilient.
The techniques and best practices discussed are essential for developing high-quality software. Consistent application of these methods will lead to improved performance, enhanced maintainability, and increased reliability, ultimately resulting in a superior user experience.