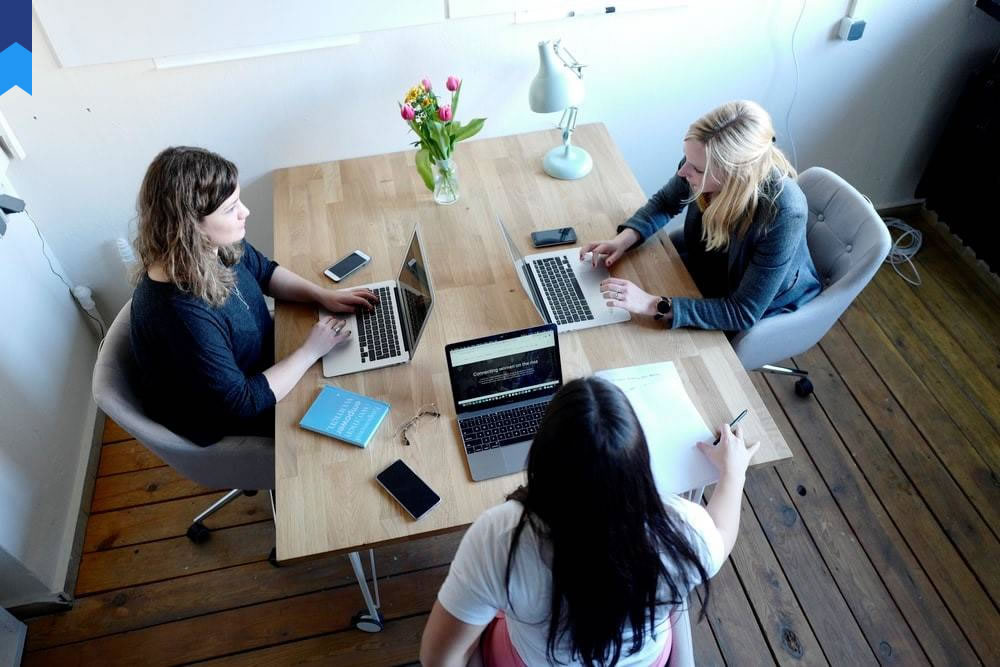
Transform Your Flutter Apps Through Advanced Dart Techniques
Dart, Google's programming language, powers the increasingly popular Flutter framework. While many resources focus on introductory Dart concepts, mastering advanced techniques is crucial for building high-performance, scalable, and maintainable Flutter applications. This article delves into several key areas, offering practical strategies to elevate your Flutter development prowess.
Advanced Dart Generics
Generics are a powerful tool for writing reusable and type-safe code. Beyond the basics, understanding advanced concepts like variance (covariance, contravariance, and invariance) is crucial for creating flexible and efficient code. Consider a scenario where you have a list of animals and a list of mammals. Since all mammals are animals, you might expect to be able to use a function designed for a list of animals on a list of mammals. This is where covariance comes in – allowing you to use a more specific type where a more general type is expected. Contravariance works the opposite way – using a more general type where a more specific type is expected, useful for functions that take types as arguments and process them. Invariance, on the other hand, strictly restricts type substitution, ensuring absolute type safety. Understanding and effectively applying these concepts can prevent many runtime errors and improve code clarity. Case study 1: Implementing a generic data repository with varying data access layers will demonstrate how variance facilitates cleaner code. Case study 2: Building a generic caching system that supports various data types illustrates the practical application of invariance.
For example, consider a generic function that finds the maximum element in a list. If the function is written using covariance, it can accept a List
Furthermore, exploring bounded type parameters allows for finer control over generic types. For instance, you might create a generic function that only accepts numerical types, ensuring type consistency across operations. This is achieved by setting bounds on the generic type parameter, for example, `T extends num`. Case study 3: Creating a generic sorting algorithm that works with various comparable types highlights the power of bounded type parameters. Case study 4: Designing a generic UI component that accepts various data models showcases the versatility of generics in Flutter UI development. The use of advanced generics minimizes the need for runtime checks, improving application performance and stability. A robust understanding of these features is essential for building scalable, efficient and maintainable code. Using Generics effectively can dramatically reduce boilerplate code and improve overall code readability and maintainability. Many experts suggest making generics a core part of any large-scale project to minimize future refactoring.
Advanced techniques like type inference and generics' interplay with other features like extension methods, and mixins, expand their capabilities significantly. Careful consideration of these interactions will lead to more elegant solutions and a better overall code structure. The effective application of Dart generics contributes towards cleaner, more maintainable code, and reduces the potential for runtime errors.
Asynchronous Programming with Streams and Futures
Dart’s asynchronous capabilities are vital for handling network requests, user input, and other time-consuming operations without blocking the main thread. While Futures are commonly understood, mastering Streams for handling sequences of asynchronous events is crucial for building responsive applications. A common scenario is fetching a large dataset from a remote server. Using a Stream allows you to process data in chunks as it arrives, preventing the application from freezing while awaiting the entire dataset. This approach improves user experience significantly. This contrasts sharply with using a Future, which would only deliver the complete result once, potentially causing delays.
Streams allow for reactive programming, enabling dynamic updates to the UI based on incoming data. Case study 1: Building a real-time chat application using Streams to handle incoming messages demonstrates the power of reactive programming. Case study 2: Creating a stock ticker application that displays live stock prices using a Stream shows how to update UI elements dynamically and efficiently. In contrast, a non-stream-based approach would require constant polling, creating unnecessary overhead. Experts recommend using Streams whenever dealing with continuous asynchronous data streams, instead of employing a cumbersome polling method.
Another aspect of asynchronous programming is error handling. Robust error handling is vital for preventing application crashes and providing informative error messages. Using `try-catch` blocks with Futures and Streams ensures that errors are gracefully handled, preventing unexpected application termination. Case study 3: Implementing a robust network request handling mechanism using asynchronous programming illustrates effective error handling practices. Case study 4: Developing a resilient data processing pipeline that recovers from transient failures showcases the importance of error handling in handling large data sets. In both scenarios, proper error handling significantly improves the application's reliability and user experience.
Furthermore, exploring advanced stream transformations like `map`, `where`, `asyncMap`, `reduce`, and `expand` allows for sophisticated data processing within the stream pipeline, without the need to fetch all data at once. This approach not only optimizes performance but also simplifies code. Combining Streams and Futures effectively with error handling allows developers to create robust and responsive applications that provide a seamless user experience. Efficient handling of asynchronous operations remains crucial for the performance and reliability of any application.
Effective Use of Isolates for Parallel Processing
Dart's isolate feature allows for true parallelism by running code in separate threads. This capability is vital for computationally intensive tasks, preventing the main thread from being blocked and ensuring responsiveness. Typical scenarios include image processing, complex calculations, and cryptographic operations. Utilizing isolates can dramatically improve the application's performance, particularly on multi-core processors. By offloading heavy tasks to isolates, developers can maintain a responsive UI even when handling complex operations.
Communication between isolates is handled through message passing, using ports. This mechanism allows for controlled data exchange between the main isolate and worker isolates. Case study 1: Implementing a background task for processing large images using isolates to avoid UI freezes demonstrates efficient task management. Case study 2: Building a computationally intensive algorithm that utilizes isolates to parallelize operations showcases how isolates can boost performance. Without isolates, these tasks would significantly impact the main thread, leading to poor user experience.
Careful design of message passing is crucial for efficient communication between isolates. Avoid sending large amounts of data through ports as this can introduce overhead. Instead, consider sending smaller, more manageable chunks of data. Case study 3: Optimizing communication between isolates by employing efficient data structures demonstrates how to minimize overhead. Case study 4: Implementing a mechanism to handle large data transfers between isolates shows how to manage substantial data flows efficiently. Using efficient techniques for message passing improves the responsiveness of the application even during heavy operations.
Moreover, understanding isolate lifecycle management and proper resource cleanup are important for preventing memory leaks and maintaining application stability. Ensuring isolates are properly terminated and their resources released is crucial for long-running applications. Effective use of isolates allows for highly efficient concurrency without jeopardizing UI responsiveness. Expert opinions strongly advocate for the use of isolates for tasks that can be parallelized to significantly improve app performance and user experience.
Working with the Dart Package Ecosystem
Leveraging the rich Dart package ecosystem is essential for efficient development. Choosing the right packages for specific tasks can drastically reduce development time and improve code quality. However, careful evaluation of package quality, security, and maintenance is crucial to prevent potential issues.
Understanding package dependencies and managing conflicts is key to a smooth development process. Using a package manager like pub greatly simplifies dependency management, allowing developers to easily include and update packages. Case study 1: Building an application that utilizes multiple packages to demonstrate efficient project structuring highlights the benefits of package management. Case study 2: Managing dependency conflicts within a Flutter project shows how to resolve conflicts and maintain project stability.
Careful consideration of package security is crucial to prevent vulnerabilities. Always check the package's reputation and security practices before integrating it into a project. Keeping packages up-to-date is essential to patch security flaws and benefit from new features and bug fixes. Case study 3: Integrating a secure and well-maintained package into a project demonstrates safe package usage. Case study 4: Addressing security vulnerabilities within a package demonstrates the importance of vigilant package management.
Additionally, understanding how to contribute to open-source packages or create your own packages enhances development skills and creates reusable components. Contributing back to the community promotes collaboration and improves the quality of existing packages. Effective utilization of the Dart package ecosystem enables rapid development, increased code reusability, and fosters collaboration within the development community.
Leveraging Dart's Metaprogramming Capabilities
Dart's metaprogramming capabilities, specifically using mirrors and annotations, offer powerful ways to generate code, modify behavior, and extend the language's functionalities. However, metaprogramming should be used judiciously, as it can increase code complexity if not implemented carefully.
Mirrors allow runtime inspection of code structure. This capability is useful for generating code based on reflections, performing runtime type checking, or building dynamic code generation tools. Case study 1: Creating a code generator that produces boilerplate code from annotations demonstrates the power of metaprogramming. Case study 2: Building a runtime type validation tool showcases the use of mirrors for improved code robustness.
Annotations provide a way to add metadata to code elements, enabling compile-time analysis and code generation. Annotations can be used for various purposes, including code documentation, generating boilerplate code, or performing code transformations. Case study 3: Using annotations to generate REST API clients demonstrates a practical application of metaprogramming. Case study 4: Implementing a dependency injection framework using annotations highlights the effective use of annotations for streamlining development.
Effective metaprogramming requires a deep understanding of Dart's runtime and compile-time behaviors. Improper usage can lead to performance issues or code fragility. Using metaprogramming judiciously improves the efficiency of development and enhances code structure. Advanced metaprogramming techniques, if implemented correctly, can lead to cleaner, more concise code, while careless implementation can severely impact readability and maintenance.
Conclusion
Mastering advanced Dart techniques is crucial for building robust, scalable, and performant Flutter applications. This exploration of generics, asynchronous programming, isolates, package management, and metaprogramming offers a pathway to enhancing your Dart proficiency. By implementing these advanced concepts, developers can improve application performance, enhance maintainability, and unlock new levels of productivity in their Flutter projects. Continuous learning and exploration of Dart’s features are key to staying ahead in the ever-evolving world of mobile application development. Remember, thoughtful application of these techniques ensures both efficiency and clarity in your code. Focusing on code readability and maintainability is just as important as leveraging the power of these advanced features.