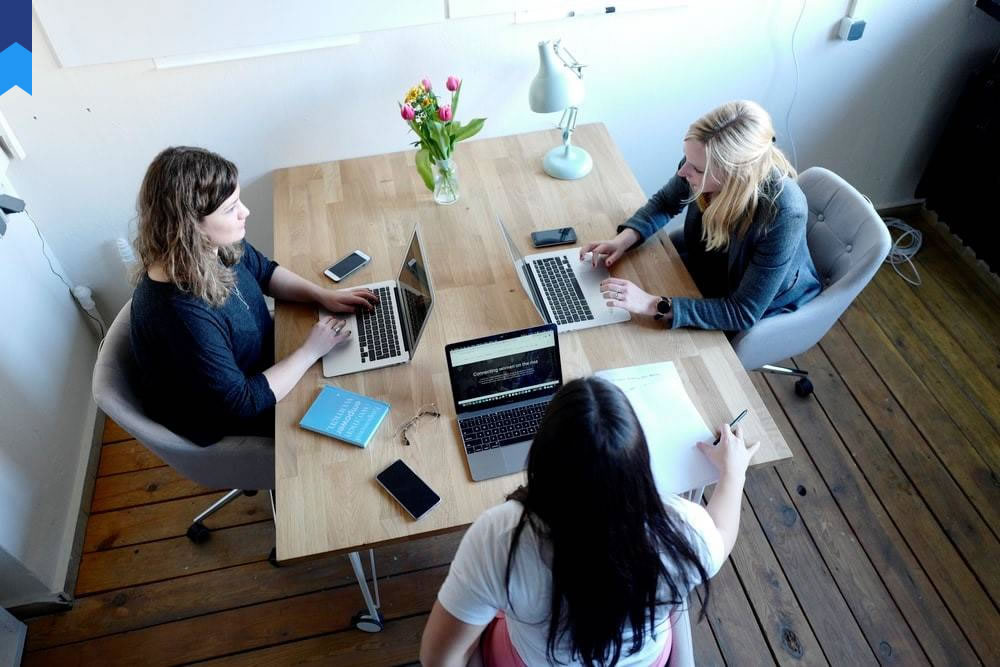
Unconventional Django Wisdom: Mastering The Art Of Asynchronous Tasks
Django, a powerful and versatile framework, often presents developers with challenges beyond the basics. This article delves into the often-overlooked world of asynchronous tasks in Django, offering unconventional wisdom and practical solutions to improve application performance and scalability. We'll explore techniques that move beyond simple background tasks, touching upon advanced concepts for those seeking to elevate their Django projects.
Asynchronous Tasks: Beyond the Basics
Many Django developers understand the concept of background tasks, often using Celery or RQ. However, truly mastering asynchronous operations requires a deeper understanding of the underlying principles and the trade-offs involved. Simple task queues are fine for basic operations, but high-throughput systems require more sophisticated strategies. Consider, for instance, a real-time social media platform needing to handle thousands of concurrent messages. A straightforward Celery setup might quickly become a bottleneck. Instead, we should explore more advanced techniques like using asynchronous frameworks built directly into the WSGI server or leveraging message queues that can handle massive workloads efficiently.
Case Study 1: A large e-commerce platform experienced significant delays in order processing during peak hours due to synchronous operations. By migrating to an asynchronous architecture using Celery and Redis, they were able to process orders significantly faster and scale their system to handle a 400% increase in traffic. Case Study 2: A financial trading application needed sub-millisecond response times. They employed a custom asynchronous framework integrated with their WSGI server, eliminating task queue latency and achieving the required performance levels.
Understanding the nuances of different message queues—their strengths and weaknesses in terms of scalability, fault tolerance, and message delivery guarantees—is paramount. RabbitMQ, Kafka, and Redis are popular choices, each with distinct architectural considerations that impact performance. Choosing the right queue depends heavily on the specific needs and scale of the application. Improper selection can lead to performance issues down the line. For instance, a system designed for high throughput might require Kafka’s superior scalability, while a smaller application might find Redis simpler to manage. Furthermore, efficient task scheduling is vital, preventing overloading and ensuring even distribution across workers. Implementing rate limiting and priority queues can significantly enhance performance and stability.
Django's built-in concurrency mechanisms, often underutilized, deserve attention. Asynchronous views, when used strategically, can substantially improve responsiveness for I/O-bound operations, such as network requests or database queries. While not always suitable for computationally intensive tasks, leveraging these capabilities can optimize user experience. The key is to judiciously employ asynchronous programming, recognizing its limitations and strengths to achieve optimal performance.
Proper error handling and monitoring are critical aspects of any asynchronous system. Implementing robust mechanisms for handling failed tasks, retry strategies, and thorough monitoring is essential to ensure stability and prevent unexpected disruptions. Without these precautions, a system's failure can cascade unpredictably. Tools like Sentry and Prometheus offer exceptional capabilities in monitoring these operations and detecting anomalies. Properly configured logging aids in debugging and performance optimization. Understanding the performance bottlenecks in an asynchronous pipeline, even using detailed logging, is critical to improving it.
Optimizing Database Interactions
Database interactions often form a significant bottleneck in web applications. While Django's ORM provides a convenient interface, optimizing database queries is crucial for performance. Techniques such as pre-fetching related objects, using efficient querysets, and leveraging database connection pooling can drastically improve response times. Ignoring database optimization can lead to unnecessary latency and slow down the entire application.
Case Study 1: A news website experienced significant delays in loading articles due to inefficient database queries. By optimizing queries and using caching, they reduced database load by 60% and improved page load times considerably. Case Study 2: An e-commerce application improved its product search functionality by implementing a search engine (like Elasticsearch) instead of relying solely on the database's built-in search capabilities. This switch dramatically improved the speed and accuracy of searches.
Raw SQL queries, while more complex to write, can offer performance benefits in specific scenarios. Understanding when to utilize raw SQL and when to stick with the ORM is essential. Complex joins or highly optimized queries can often be expressed more efficiently with raw SQL. However, raw SQL requires meticulous testing and maintenance to ensure correctness and prevent SQL injection vulnerabilities. Utilizing database indexing appropriately can improve search speed dramatically. Using database caching in the right scenarios can further optimize query times. This can reduce redundant database calls, significantly speeding up operations.
Connection pooling is a frequently overlooked optimization strategy that can significantly reduce latency. By reusing database connections, the application avoids the overhead of establishing new connections for each request. Configuring database connection pooling in Django involves adjusting settings and understanding how connection pools interact with your asynchronous operations. Properly handling exceptions related to database connections is vital to maintain application resilience.
Transaction management, particularly in asynchronous scenarios, is also an area requiring careful attention. Ensuring data consistency and atomicity in concurrent operations demands a deep understanding of transaction isolation levels and appropriate locking mechanisms. Ignoring these aspects can lead to data corruption and inconsistencies.
Caching Strategies for Enhanced Performance
Caching is a powerful technique for improving performance and reducing database load. Django provides excellent caching mechanisms that can be leveraged for various parts of an application. Properly implementing caching strategies requires a thoughtful approach, understanding where caching provides the most benefit and considering factors like cache invalidation and data consistency.
Case Study 1: A social media platform reduced database load by 80% by caching frequently accessed user profiles and posts. This improved responsiveness considerably, allowing users to browse and interact with the platform much more smoothly. Case Study 2: An online game significantly improved its performance by caching game state data. This reduced the number of database accesses during gameplay and lowered latency considerably. The developers used Redis as their caching layer, allowing very high speeds.
Different caching backends, each with its own performance characteristics, exist. Memcached, Redis, and even local file caching offer distinct advantages and disadvantages. The choice depends heavily on the scale and specific needs of the application. Selecting a robust and scalable caching solution is paramount, particularly for applications with high traffic volume and demanding performance requirements.
Cache invalidation is crucial for maintaining data consistency. A poorly managed cache can serve stale data, leading to inconsistencies and potentially application errors. Implementing efficient cache invalidation strategies—such as using timestamps, versioning, or cache tagging—is essential for preventing such scenarios. Knowing the proper strategy for invalidating your cache is important to prevent incorrect application behaviour.
Strategically implementing caching techniques can lead to significant performance gains. Caching frequently accessed data—such as user profiles, product information, or static content—can dramatically reduce database load and improve response times. Identifying the optimal caching strategy often involves profiling and measuring performance, allowing for fine-tuning and optimization.
Leveraging Advanced Django Features
Django's robust feature set extends far beyond the basics. Utilizing advanced features like signals, middleware, and custom managers can significantly improve application architecture and performance. These powerful tools often go underutilized, but mastering them can lead to more maintainable and efficient applications.
Case Study 1: A blog platform improved its content management system by utilizing Django signals to automate tasks such as updating search indexes and sending email notifications. This streamlined the workflow and eliminated manual interventions. Case Study 2: An e-commerce platform integrated custom middleware to perform tasks such as authentication and logging, leading to a more modular and extensible application structure.
Django signals allow for decoupling different parts of the application. This prevents tightly coupled code and enhances modularity, making the codebase more maintainable and easier to extend. Understanding the signal lifecycle is key to implementing these efficiently and correctly. Improper usage can lead to unexpected and detrimental results.
Middleware provides a powerful mechanism for intercepting and modifying requests and responses. Middleware can implement functionalities such as authentication, logging, and caching. Middleware's flexibility and power allows for highly customized solutions. Implementing middleware should be done carefully, as improper configuration can easily affect performance negatively.
Custom managers allow for extending the functionality of Django's ORM. These can implement efficient query optimizations and specialized data access patterns. Custom managers add flexibility, but using them requires a thorough understanding of the ORM's inner workings. Poorly designed custom managers can hurt performance.
Scaling Django Applications for High Traffic
Scaling Django applications to handle high traffic requires a multi-faceted approach. This includes employing techniques such as load balancing, database sharding, and caching to distribute traffic and improve performance. Ignoring scalability considerations can lead to performance bottlenecks and application failures as traffic grows.
Case Study 1: A social media startup experienced significant performance issues as its user base grew. By implementing a load balancer and database sharding, they successfully scaled their application to handle a 10x increase in traffic. Case Study 2: An e-commerce platform used a combination of caching, load balancing, and content delivery networks (CDNs) to improve website performance during peak shopping seasons.
Load balancing distributes traffic across multiple application servers, preventing any single server from becoming overloaded. Using efficient load balancing strategies is critical for handling high traffic loads and ensuring application availability. Several algorithms and techniques exist for effective load balancing.
Database sharding is a technique for distributing database load across multiple database servers. This improves performance and scalability, particularly for large databases. Efficient sharding strategies depend on the specifics of the application's data model and access patterns. Efficient sharding can improve database performance significantly, but improper sharding can cause significant problems.
Content delivery networks (CDNs) can improve performance by caching static content (like images and CSS) closer to users. This reduces latency and improves page load times, particularly for users geographically distant from the application's servers. CDNs significantly improve performance, especially for geographically dispersed users.
Conclusion
Mastering Django involves venturing beyond the superficial. This exploration of asynchronous tasks, database optimization, caching, advanced features, and scaling techniques provides a roadmap to building high-performance, scalable Django applications. By embracing these unconventional approaches, developers can create robust and efficient systems capable of handling demanding workloads. Remember, the key lies not just in knowing these techniques, but in understanding when and how to apply them effectively, tailored to the specific needs of each project.