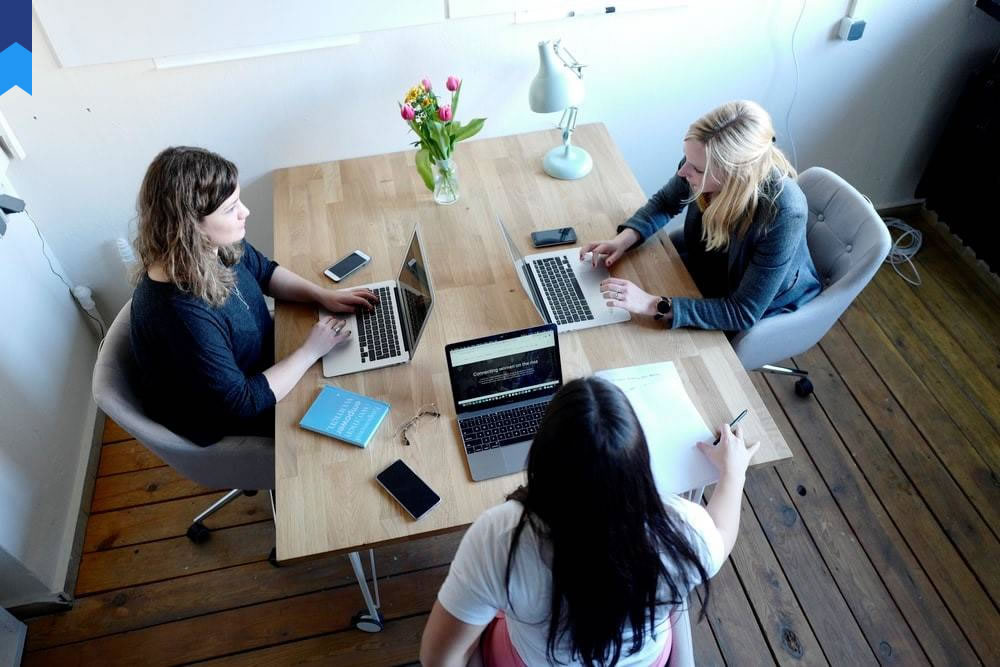
Unconventional Kotlin Wisdom: Mastering The Art Of The Unexpected
Kotlin, with its concise syntax and robust features, has become a favorite among Android developers. But beyond the basics lies a world of unconventional techniques and practices that can significantly enhance your coding skills and project efficiency. This article delves into these often-overlooked aspects, offering a fresh perspective on Kotlin programming.
Delegated Properties: Beyond the Obvious
Delegated properties are a powerful Kotlin feature often underutilized. They allow you to delegate the implementation of a property to another object, simplifying code and improving readability. Instead of writing boilerplate getters and setters, you can leverage delegated properties to handle complex logic or external data sources efficiently. Consider a scenario where you need to persist user preferences. A simple delegated property using `PreferenceDelegate` can handle the read/write operations to your preferences file, abstracting away the complexities. Case study one: Imagine managing application-wide settings. Using a delegated property, the settings can be stored and retrieved from an external file or database, without cluttering your main class.
Another common use is lazy initialization. If you have a property that is computationally expensive to create and only needed sometimes, a lazy delegated property will ensure it's only created when first accessed, boosting performance. Case study two: A game might have heavy assets. They would only load when the game level requires them. This avoids loading times.
Furthermore, delegated properties find use in observer patterns, where changes to a property automatically trigger actions in other parts of your application. This clean separation of concerns promotes better code organization and maintainability. Delegated properties allow a smooth implementation of reactive programming patterns, making code more responsive and dynamic. The possibilities extend to managing complex state and even dependency injection.
For instance, consider scenarios involving caching or network requests. A delegated property can seamlessly handle fetching data, caching results and updating the UI when new information is available. Effective use of delegated properties can lead to cleaner, more maintainable, and high-performance code. The key is to understand the different types of delegated properties and how to choose the appropriate one for your specific needs. This helps avoid unnecessary complexity and enhances overall coding efficiency.
Modern applications often require asynchronous operations. Managing asynchronous calls efficiently is crucial. Kotlin's coroutines offer an elegant way to handle asynchronous programming, simplifying complex code and enhancing readability. Coroutines, paired with delegated properties, provides a powerful combination for managing asynchronous tasks within your application.
Efficient error handling is a cornerstone of robust software. By handling errors in a structured way, developers can safeguard the stability of their application. Delegated properties offer an ideal method for streamlining error handling, promoting code clarity and improving maintainability.
Coroutine Scope and Context: Unleashing Asynchronous Power
Kotlin coroutines offer a powerful way to handle asynchronous operations, but mastering their scope and context is crucial for building robust and maintainable applications. Understanding the nuances of coroutine scopes allows developers to control the lifecycle of coroutines, preventing memory leaks and ensuring proper resource management. For example, using a lifecycle-bound coroutine scope, like `viewModelScope` in Android development, guarantees that coroutines are cancelled when the associated lifecycle component is destroyed. This prevents resource leaks and improves overall application stability.
Context plays a key role in shaping how coroutines behave. A coroutine context is composed of elements such as dispatchers and job, determining which thread a coroutine runs on and how it reacts to cancellation. Selecting an appropriate dispatcher — `Dispatchers.IO` for I/O-bound operations or `Dispatchers.Main` for UI updates — is essential for optimal performance. Case study one: An image loading library uses `Dispatchers.IO` to load images from a network in the background without blocking the main thread. The result is then dispatched to the main thread via `Dispatchers.Main` for display.
Managing coroutine exceptions is another crucial aspect. Unhandled exceptions in coroutines can lead to application crashes. Proper exception handling is critical. Using `coroutineScope` or `supervisorScope` allows you to manage exceptions from child coroutines efficiently. `supervisorScope` is especially useful when the failure of one coroutine shouldn't bring down the entire application. Case study two: Imagine a shopping cart app with several items fetching prices concurrently. If one item's price cannot be fetched, it shouldn't prevent fetching the prices of other items.
The proper use of structured concurrency with coroutines prevents accidental leaks or errors. A common pattern is to launch coroutines within a `viewModelScope`, ensuring they are cancelled automatically when the ViewModel is destroyed. This promotes clean code and resource management. Understanding the context, scope, and exception handling capabilities of coroutines is vital for building robust applications. This leads to better performance and more resilient code.
Another significant advantage of using coroutines is their ability to handle cancellation. This is crucial in scenarios where operations might take a long time or the need arises to abort an operation before completion. With coroutines, you can gracefully cancel operations, preventing resource waste and improving user experience. Correctly managing coroutine cancellation requires a clear understanding of the `Job` and its cancellation methods.
Advanced concepts like `CoroutineExceptionHandler` allow you to handle exceptions in a central location, making debugging and error reporting easier. By leveraging these features, developers can improve the robustness and reliability of their applications. A structured approach to coroutine management is essential for building high-quality applications.
Sealed Classes: Enforcing Type Safety
Sealed classes in Kotlin provide a powerful mechanism for representing restricted sets of types, improving type safety and code readability. They are particularly useful when dealing with states, events, or different types of data that need to be handled in a controlled manner. By restricting the possible subtypes, you can create more robust and less error-prone code. Case study one: In a UI state management system, a sealed class can represent different states, such as loading, success, and error. This enforces that all possible states are handled explicitly, improving code quality.
Sealed classes also enhance code readability by making the possible types explicit. This eliminates ambiguity and improves the understandability of the codebase. The compiler enforces that all subtypes are handled, leading to fewer runtime errors. Case study two: An API response could be a success with data, an error with a message, or loading state. The sealed class ensures you must handle every possible case, avoiding unexpected behavior.
When combined with `when` statements, sealed classes provide an elegant way to perform exhaustive pattern matching. The compiler ensures that all possible subtypes are covered, preventing errors that might occur when handling different types of data. This is especially valuable when dealing with complex logic that requires handling numerous cases.
Sealed classes improve the maintainability of your codebase because modifications to the set of possible types are immediately reflected in all parts of the code where the sealed class is used. This reduces the risk of errors introduced by changes in the data structure. Understanding and using sealed classes effectively is a hallmark of robust Kotlin programming.
Beyond their benefits in state management, sealed classes can be used effectively in data modeling to represent different types of entities. This approach leads to cleaner and safer code compared to using traditional interfaces or abstract classes.
Sealed classes integrate well with Kotlin's other features like data classes and extensions, further simplifying the coding process. This synergistic integration simplifies code, improves readability and makes it easier to maintain.
Higher-Order Functions: Functional Programming Power
Kotlin's support for higher-order functions—functions that take other functions as arguments or return functions—allows for concise and expressive code. This functional approach enables developers to write more flexible and reusable code. For instance, a simple function to filter a list of numbers can be made reusable by passing a filter condition as an argument. This allows for filtering based on different criteria without writing multiple similar filtering functions. Case study one: A data processing pipeline can be created by chaining higher-order functions like `map`, `filter`, and `reduce`, making the code more modular and readable.
Higher-order functions foster code reuse. The same higher-order function can be used with many different functions, simplifying the process of developing versatile and maintainable applications. Case study two: An image processing library might have a function to apply various filters to images using a higher-order function that takes a filter function as an argument. This design enhances code flexibility and reduces redundancy.
Higher-order functions can simplify asynchronous operations, such as network requests, enabling developers to handle callbacks or futures in a more manageable manner. The functional style simplifies complex interactions between asynchronous operations, enhancing the code's clarity.
Kotlin's lambda expressions and function literals simplify the implementation of higher-order functions. These concise constructs allow for cleaner syntax and better code readability when working with higher-order functions. Combining higher-order functions with lambda expressions is a cornerstone of functional programming in Kotlin.
Higher-order functions improve code organization by reducing code duplication. This results in a leaner codebase, improving overall maintainability and reducing the chances of introducing bugs.
The functional programming paradigm, leveraging higher-order functions, makes code easier to test and debug. The modular nature allows for focusing testing on individual functions, making the process more efficient.
Extension Functions: Extending Functionality Without Inheritance
Extension functions in Kotlin allow you to add new functionality to existing classes without modifying their source code or using inheritance. This is a powerful tool for improving code organization and maintainability. For example, you can add a utility function to a standard Kotlin class like `String` to easily perform common tasks without creating a subclass. Case study one: Extending the String class with a function to reverse a string adds functionality without modifying the original class.
Extension functions enhance code readability and conciseness. They provide a more intuitive syntax for adding features to existing classes. Case study two: Adding a function to the `List` class to efficiently find the maximum value eliminates the need for manual iterations, improving code readability and efficiency.
Extension functions can be used to implement domain-specific languages (DSLs) in Kotlin. They make the syntax cleaner and more tailored to your specific needs. This leads to more concise and expressive code within the specific domain.
Extension functions are especially useful when working with third-party libraries. You can extend the functionality of existing classes without modifying the library's code, preventing conflicts and maintaining compatibility.
Proper use of extension functions promotes code modularity and enhances the maintainability of projects. It leads to a cleaner and more organized codebase, reducing the potential for errors and improving collaboration.
Extension functions also contribute to improved code reusability by providing a concise way to add common functionalities to various classes without repeating code. This reduces redundancy and promotes efficiency.
Conclusion
Kotlin's power extends far beyond its basic syntax. Mastering advanced features like delegated properties, coroutines, sealed classes, higher-order functions, and extension functions unlocks a realm of elegant, efficient, and robust code. By embracing these unconventional approaches, developers can elevate their Kotlin skills, building more maintainable and high-performance applications. This deeper understanding transcends simple tutorials and guides to truly harness the potential of Kotlin programming. The key is consistent practice and exploration, venturing beyond the typical examples to understand the nuances and possibilities of these advanced features. This allows developers to create innovative and high-quality applications, showcasing the true capabilities of Kotlin.