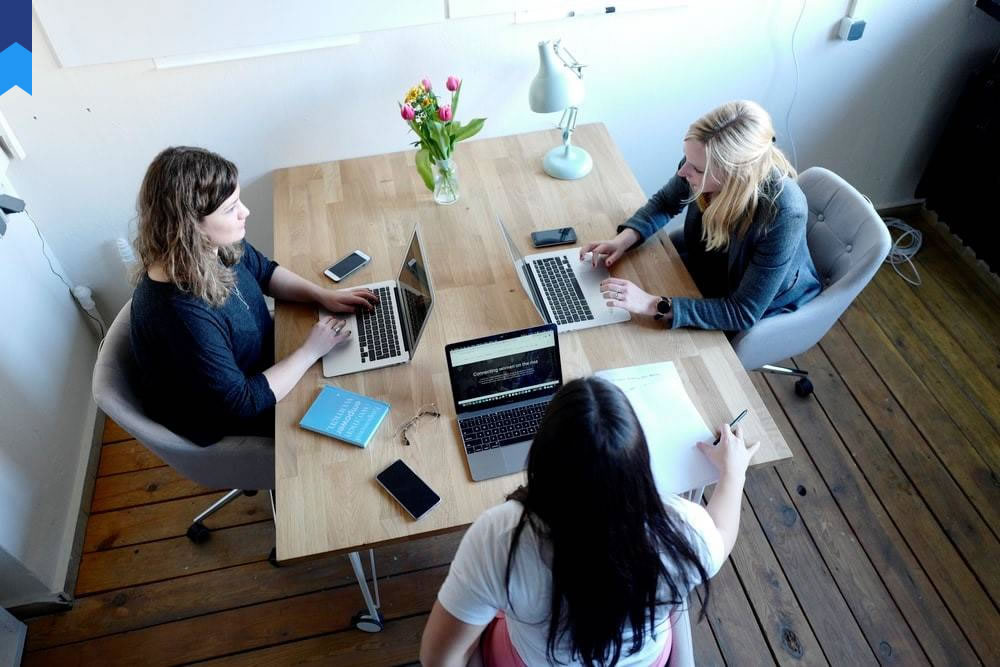
Unconventional Wisdom: A Fresh Take On Arduino Programming
Arduino programming, often perceived as a straightforward path to embedded systems development, actually holds a treasure trove of unconventional techniques and approaches. This article dives beyond the basics, exploring innovative strategies that can unlock the full potential of your Arduino projects.
Mastering Interrupt Handling for Enhanced Responsiveness
Interrupt handling is a cornerstone of efficient Arduino programming, allowing your microcontroller to respond to external events without halting the main program flow. Conventional approaches often involve simple interrupt service routines (ISRs) that handle a single task. However, more sophisticated strategies, such as nested interrupts and interrupt prioritization, can greatly enhance system responsiveness, especially in complex projects. For example, consider a project integrating multiple sensors with varying priorities: a high-priority emergency stop button might interrupt a lower-priority data acquisition routine. Efficient interrupt handling ensures that critical events are addressed promptly, without compromising the functionality of other parts of the system. Case study 1: A robotic arm project utilizing interrupts to handle both encoder feedback and operator commands simultaneously demonstrated a significant increase in speed and precision compared to a non-interrupt-driven approach. Case study 2: A multi-sensor environmental monitoring system employed prioritized interrupts to guarantee the timely logging of critical temperature and pressure readings, even during high-volume data acquisition from other sensors. Efficient handling of interrupts necessitates careful design and consideration of timing constraints. Improperly configured interrupts can lead to unexpected behavior, including lost data and system instability. Understanding interrupt latency, context switching overhead, and potential conflicts between different interrupt sources are critical aspects for successful implementation. Advanced techniques like interrupt chaining, where one interrupt triggers another, can be particularly useful for complex systems.
Further expanding on interrupt handling, consider the use of different interrupt sources. The Arduino platform provides various pins capable of triggering interrupts, from external hardware triggers to internal timers. Selecting the appropriate interrupt source depends on the specific application. Utilizing timers as interrupt sources allows for precise timing of events, enabling applications requiring periodic actions or synchronization with external systems. For instance, a motor control system using Pulse Width Modulation (PWM) can significantly benefit from timer interrupts for precise control. Another method involves using interrupts to manage asynchronous communication protocols like I2C or SPI, providing a more responsive and efficient data exchange. Furthermore, the use of interrupt vector tables for managing multiple interrupt sources should be considered for large-scale projects. By carefully managing interrupt sources, programmers can drastically improve the responsiveness and efficiency of their Arduino-based systems.
Beyond the fundamentals of interrupt handling, exploring advanced concepts like interrupt coalescing further optimizes resource usage. Interrupt coalescing combines multiple interrupts into a single interrupt event, reducing the overhead associated with processing numerous individual interrupts. This technique becomes invaluable in scenarios with high-frequency interrupt generation, such as applications involving fast-moving sensors. This reduces CPU load, resulting in a significant performance boost and less energy consumption. Similarly, using techniques for optimizing interrupt routines themselves, such as minimizing code within the ISR and utilizing efficient data structures, is crucial for achieving maximum performance. Advanced techniques like preemptive multitasking, although challenging in resource-constrained environments, can greatly improve responsiveness and efficiency when implemented correctly.
In summary, mastering interrupt handling in Arduino programming requires a deep understanding of the underlying hardware and software interactions. Through careful design, selecting optimal interrupt sources, and employing advanced techniques like coalescing and efficient ISR optimization, developers can create highly responsive and efficient Arduino-based systems. The benefits include improved performance, greater reliability, and reduced power consumption.
Unlocking the Power of State Machines
State machines provide an elegant and powerful way to manage the complex behavior of Arduino projects. Instead of using lengthy `if-else` structures, a state machine defines the system's possible states and transitions between them, greatly enhancing code readability and maintainability. A simple example might be a traffic light controller: the system transitions between green, yellow, and red states based on a timer. This structured approach simplifies debugging and allows for systematic expansion of project functionality. Case study 1: A complex robotic control system used state machines to manage the various stages of a robotic arm's movement, resulting in improved reliability and easier troubleshooting. Case study 2: A home automation system utilized state machines to track the status of multiple devices and efficiently manage power consumption. Efficient state machine design involves clearly defining the states and transitions, using well-chosen state variables, and ensuring that each state has a clear entry and exit condition. This reduces the likelihood of errors and increases code maintainability.
Beyond basic state machines, hierarchical state machines (HSMs) allow for modeling more complex systems by nesting state machines within each other. This modular approach helps manage the complexity of large projects by breaking them down into smaller, more manageable units. Each sub-machine can represent a distinct part of the system, allowing developers to focus on a specific task and integrate them seamlessly into the larger system. This hierarchical approach enhances readability, making complex systems more approachable and easier to maintain. The use of state machine diagrams, such as statecharts, is invaluable for visualizing the system's states and transitions, facilitating both design and debugging. These diagrams provide a visual representation of the program logic, helping identify potential issues and inconsistencies early in the development process. Moreover, leveraging state machine libraries can streamline development, providing pre-built functions and utilities, further improving efficiency and productivity.
Furthermore, the combination of state machines with other advanced techniques, like event-driven programming and reactive programming, enhances the system’s responsiveness and efficiency. Event-driven programming allows the state machine to react to external events, such as button presses or sensor readings, triggering transitions between states. Similarly, reactive programming, focusing on data flows and transformations, can be effectively integrated with state machines, allowing for more dynamic and responsive systems. Advanced state machine implementations utilize features like guards and actions, enhancing expressiveness and functionality. Guards add conditions to transitions, enabling more nuanced control over state changes. Actions allow for executing specific operations during transitions, streamlining tasks like data logging or actuator control. This nuanced approach enhances the precision and flexibility of state machine design. The choice between different state machine implementations, such as Moore machines and Mealy machines, depends on the application's specific requirements and the level of complexity. Each type offers unique advantages and disadvantages, affecting the system's behavior and the design tradeoffs involved.
In conclusion, state machines are a powerful tool for creating robust and maintainable Arduino projects. By adopting this paradigm, developers can drastically improve code organization, simplify debugging, and create scalable and easily expandable applications. Employing advanced techniques like hierarchical state machines and incorporating event-driven and reactive programming further unlocks the potential of this approach. The benefits extend beyond mere code organization, impacting the overall efficiency, reliability, and maintainability of the system.
Efficient Memory Management Techniques
Arduino's limited memory resources pose a significant constraint, requiring careful attention to memory management practices. Conventional wisdom often overlooks the subtleties of memory allocation and deallocation, leading to unexpected behavior and crashes. Understanding the Arduino memory architecture, including RAM and Flash limitations, is crucial for optimizing memory usage. Employing dynamic memory allocation judiciously, avoiding unnecessary memory fragmentation, and releasing allocated memory promptly are critical strategies. Case study 1: A project that attempted to process large images directly in RAM experienced frequent crashes; optimizing the image processing algorithm to use external storage (SD card) solved the memory issues. Case study 2: A data logging application initially used arrays of fixed size, leading to wasted memory when the data quantity varied; employing dynamic memory allocation with linked lists greatly improved efficiency. Optimized data structures play a critical role in managing memory effectively. Choosing the right data structure for a given application can drastically reduce memory consumption. For example, using linked lists instead of arrays when the data size is unknown, or implementing efficient search algorithms to minimize memory usage while maintaining quick access are essential. This also minimizes memory fragmentation, a frequent cause of memory exhaustion. Analyzing and profiling memory usage within a program using tools and techniques provided by the Arduino IDE enhances the understanding of memory-intensive parts and aids in optimization strategies.
Expanding on efficient memory management, programmers should consider utilizing techniques such as memory pooling. Memory pooling pre-allocates a set of memory blocks, providing a readily available pool of memory for frequently used objects, thereby avoiding frequent heap allocation and deallocation, which significantly reduces fragmentation and increases performance. This is particularly beneficial in systems with frequent object creation and destruction. Additionally, optimizing the use of variables and data types is equally important. Using smaller data types whenever possible significantly reduces memory footprint. Careful consideration of variable scope and lifetime also plays a significant role. Utilizing local variables instead of global ones when possible minimizes global memory usage and can also improve code organization. Furthermore, adopting code optimization techniques further reduces the application's memory footprint. Minimizing unnecessary variable declarations, avoiding redundant calculations, and using efficient algorithms can significantly conserve resources. Compiling the code with optimization flags can also enhance the efficiency of memory usage. This approach improves memory management by effectively utilizing the available resources. Careful attention to these details is critical for effective memory management.
Furthermore, employing techniques for data compression and encoding can significantly reduce the memory footprint, especially when handling large datasets. Using methods like Run-Length Encoding (RLE) or Huffman coding can efficiently represent data, minimizing the storage space required. Moreover, leveraging external memory, such as SD cards or EEPROM, for storing large datasets frees up valuable RAM. This approach allows the program to handle significantly larger datasets without encountering memory limitations. In addition to optimizing data structures and algorithms, implementing efficient memory allocation strategies is key. Utilizing techniques like first-fit and best-fit allocation algorithms, while considering the fragmentation aspects, can minimize memory fragmentation and improve overall performance. These algorithms help organize memory allocation, reducing memory waste and increasing efficiency. These strategies are particularly effective in situations where dynamic memory allocation is heavily used. Understanding and using these techniques effectively are crucial aspects of optimized memory management.
In conclusion, efficient memory management is a critical aspect of Arduino programming. By carefully selecting data structures, optimizing data types and algorithms, and leveraging external memory and advanced techniques, developers can overcome memory limitations and create more robust and efficient applications. These approaches are paramount in maximizing resource utilization and ensuring application stability within the constraints of Arduino’s limited memory.
Leveraging Libraries and Frameworks
Arduino's vast ecosystem of libraries provides pre-built functions and modules for a wide range of tasks, offering significant time savings and reducing development effort. However, selecting appropriate libraries and understanding their limitations is critical. Conventional approaches often involve searching for the "best" library, but a more effective strategy involves evaluating multiple libraries based on their suitability for the specific project's requirements. This includes factors such as size, efficiency, and compatibility with other libraries used in the project. Case study 1: A project involving multiple sensors utilized separate libraries for each sensor, leading to compatibility issues; refactoring the code to use a single library simplified the project and improved stability. Case study 2: A project initially used a large, feature-rich library, impacting performance; switching to a smaller, more focused library significantly improved efficiency. Careful selection of libraries enhances overall performance, maintainability, and reduces the possibility of conflicts within the application. The judicious use of libraries can improve the developer's overall efficiency, enabling faster development cycles. Understanding the tradeoffs between the different libraries is crucial for successful integration. Factors such as memory footprint, performance, and ease of use need to be considered. The benefits of well-integrated libraries are significantly increased productivity and enhanced software quality. This approach minimizes code redundancy and increases project stability.
Expanding on the use of libraries, developers should also consider creating their own custom libraries for frequently reused code blocks or project-specific functions. This promotes code reusability and maintainability across multiple projects. Modular design and well-documented custom libraries facilitate collaboration and improve the overall code quality. The process of creating a custom library involves encapsulating related functions and variables into a single unit. This helps to organize the code, making it easier to maintain and understand. A well-structured custom library can drastically improve the efficiency of the development process and reduce the occurrence of errors. This structured approach enhances code readability, making it easier to debug and maintain. Proper documentation and clear naming conventions within a custom library are equally critical aspects of creating robust libraries. Thorough documentation and clear naming conventions improve readability and ease of use, making the library accessible to other developers. This is an essential element for collaboration, ensuring code maintainability and easy understanding.
Moreover, the effective use of libraries also includes understanding the licensing terms associated with each library. Open-source libraries are frequently used, and it is crucial to respect the licensing conditions to avoid legal problems. Choosing libraries with licenses compatible with the project's requirements is critical. Understanding the implications of the specific license ensures that the project complies with all legal and ethical considerations. In addition, maintaining libraries and frequently checking for updates is also important. Library maintainers regularly release updates that include bug fixes, performance improvements, and new features. This ensures that the project benefits from the latest developments and is kept updated with any necessary changes. This continuous improvement ensures that the project remains functional and up to date with recent advancements.
In conclusion, leveraging libraries and frameworks is crucial for efficient Arduino programming. By carefully selecting appropriate libraries, creating custom libraries for reusable code, understanding licensing terms, and staying current with updates, developers can enhance productivity, maintainability, and reliability of their projects. Effective library management is essential for robust and successful Arduino development.
Advanced Debugging and Troubleshooting Techniques
Debugging and troubleshooting are inherent parts of Arduino development. Conventional methods often rely on serial print statements, but more advanced techniques can significantly expedite the process. Utilizing a logic analyzer or an oscilloscope to visually inspect signals can pinpoint timing issues or hardware problems that serial monitoring might miss. Case study 1: A project with intermittent failures traced to a timing issue was quickly resolved by using a logic analyzer to visualize signal timing. Case study 2: A sensor integration problem identified through visual inspection using an oscilloscope revealed a mismatch in voltage levels. These tools provide a more comprehensive view of the system's behavior, simplifying the troubleshooting process. Understanding the intricacies of hardware and software interaction is essential for effective debugging. Identifying the source of a problem often requires a combined approach of software analysis and hardware diagnostics. Visual inspection using tools such as oscilloscopes and logic analyzers adds significant value to the debugging workflow.
Expanding on advanced debugging techniques, employing debugging tools such as a dedicated debugger integrated with the Arduino IDE enables setting breakpoints within the code. Stepping through the code line by line provides a granular analysis, allowing developers to inspect variable values and program flow at every step. The use of such debugging tools drastically reduces troubleshooting time and significantly improves the overall development efficiency. The precise identification of the source of errors, using debugging tools, provides a more structured and accurate troubleshooting strategy compared to traditional guesswork approaches. This targeted approach significantly streamlines the debugging process and increases development efficiency. Leveraging the features of integrated development environments (IDEs) is crucial for successful debugging. IDEs often include advanced debugging tools and features that simplify the process. Understanding the features and functionalities of the chosen IDE is crucial for efficient debugging. The systematic use of such tools improves the overall quality of the software developed.
Furthermore, implementing robust logging mechanisms within the code aids in identifying issues that might only occur under specific circumstances. Logging provides a detailed record of the program's execution, including variable values, timestamps, and sensor readings. This detailed information provides valuable insights into the program’s behavior and helps in identifying potential causes of errors. This approach is particularly useful in scenarios where the error is not consistently reproducible. Well-placed logging statements offer detailed insights into the program flow and variable states during execution. This granular-level insight into the program's execution is often essential for identifying the root cause of problems. This method becomes especially important in complex projects where several components interact with each other.
In summary, advanced debugging and troubleshooting techniques are essential for efficient Arduino development. Employing logic analyzers, oscilloscopes, dedicated debuggers, and robust logging mechanisms significantly improves the efficiency of the debugging process. The combination of these techniques ensures that errors are identified and resolved promptly, promoting robust and reliable applications. The systematic approach to debugging using these techniques yields high-quality software that functions consistently and effectively.
Conclusion
Arduino programming, while seemingly straightforward, presents opportunities for innovation and optimization beyond the basic tutorials. Mastering advanced techniques, such as refined interrupt handling, state machine implementation, meticulous memory management, strategic library utilization, and comprehensive debugging methods, transforms Arduino projects from simple demonstrations to sophisticated and robust embedded systems. By embracing these unconventional approaches, developers unlock the full potential of the Arduino platform, creating innovative and efficient solutions for a wide range of applications. The journey from basic Arduino programming to advanced techniques requires dedicated exploration and practice, but the rewards—in terms of enhanced performance, improved reliability, and greater coding efficiency—are substantial.
The exploration of advanced concepts presented throughout this article demonstrates that Arduino programming extends far beyond the introductory level. The advanced methodologies outlined offer substantial improvements in efficiency, robustness, and code maintainability. Mastering these techniques empowers developers to create more complex and sophisticated projects, moving beyond simple demonstrations to build robust and reliable embedded systems.