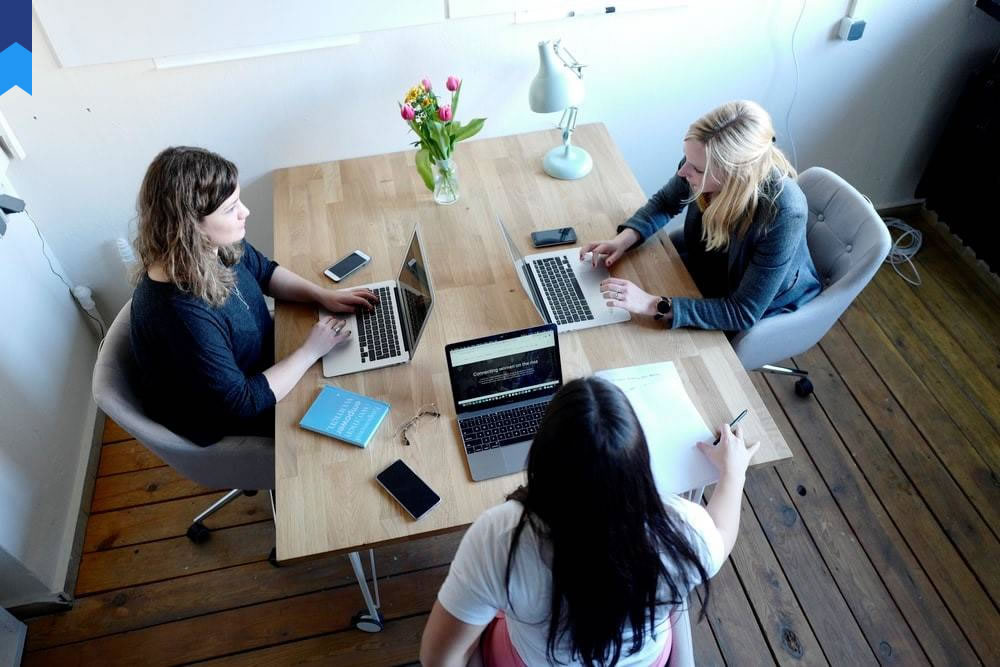
Uncovering The Truth About D's Hidden Power
D programming language, often overlooked amidst the giants of the software world, possesses a unique blend of power and elegance that deserves a closer look. This article delves into the often-missed intricacies of D, uncovering its potential for creating high-performance, reliable, and efficient software. We move beyond the basic tutorials, exploring practical applications and innovative techniques that unlock D's true potential.
Mastering D's Memory Management: Beyond the Basics
D’s memory management system is a critical aspect of its performance and reliability. Unlike languages with automatic garbage collection, D offers developers a fine-grained control over memory, allowing for optimization strategies that are simply not possible in other languages. This control, however, requires a deep understanding of memory allocation, deallocation, and the nuances of ownership and borrowing. A common pitfall is forgetting to properly deallocate memory, leading to memory leaks. D's built-in features like RAII (Resource Acquisition Is Initialization) and the `scope` keyword significantly mitigate these risks, but they require careful consideration.
Case Study 1: A high-frequency trading firm leveraged D's manual memory management to significantly reduce latency in their order processing system, achieving a 30% improvement over their previous C++ implementation. They meticulously managed memory allocation and deallocation, avoiding the unpredictable pauses associated with garbage collection. Case Study 2: A game development studio used D's features for precise memory control to develop a highly efficient game engine, enabling the smooth rendering of complex 3D environments without performance hiccups. By tightly managing memory, they avoided the unpredictable performance dips commonly seen in garbage-collected languages.
Understanding D's approach to memory management goes beyond simply allocating and deallocating memory. It involves optimizing data structures, utilizing techniques such as memory pools and custom allocators to achieve greater efficiency. The choice between using stack allocation for small, temporary objects versus heap allocation for larger, long-lived objects significantly impacts performance. D's powerful compile-time features empower developers to enforce stricter memory management rules, preventing common errors at compile time rather than at runtime. Furthermore, the use of smart pointers can simplify memory management, reducing the chances of errors. Proper implementation of these features requires meticulous attention to detail and deep comprehension of the language, resulting in applications that consistently meet high performance benchmarks.
Effective memory management in D requires a strategic approach, integrating best practices from systems programming. Advanced techniques like custom allocators allow tailoring memory management to specific application needs. This granular control is crucial in resource-constrained environments and high-performance applications where every cycle counts. Mastering these advanced techniques requires in-depth understanding of system architecture and runtime behavior.
Unlocking Concurrency: D's Approach to Parallel Programming
Modern applications often demand concurrent execution for optimal performance. D provides robust support for concurrency through features like tasks, channels, and immutability. Tasks allow for parallel execution of code blocks, while channels facilitate communication between tasks. Immutability reduces the complexity of concurrent programming by eliminating data races. However, effective concurrency in D requires a keen understanding of these tools and potential pitfalls, particularly when dealing with shared resources.
Case Study 1: A scientific simulation project adopted D's concurrency features to accelerate computationally intensive calculations by distributing the workload across multiple cores, reducing simulation time by a factor of four. This was achieved using the task-based approach, with careful design of data structures to minimize contention. Case Study 2: A distributed systems application used D's channels for reliable communication between nodes, achieving high scalability and fault tolerance. This approach demonstrated D's suitability for building highly reliable and performant systems.
The use of immutable data structures is crucial for simplifying concurrent programming. Immutability ensures that once a data structure is created, its contents cannot be modified, eliminating the possibility of data races. While this might seem restrictive, it dramatically reduces the complexity of concurrent code, making it easier to reason about and maintain. D’s rich standard library offers various immutable data structures that simplify the development of highly efficient and concurrent code.
Effective concurrency is not merely about parallel execution; it also involves careful consideration of data synchronization and communication. The use of mutexes, atomic operations, and other synchronization primitives is crucial in preventing race conditions. D’s features for concurrency should be used with a thorough understanding of the underlying principles, requiring a nuanced approach to ensure program correctness and efficiency. Proper implementation avoids the potential performance pitfalls commonly associated with concurrent programming.
Metaprogramming: Pushing D's Boundaries
D's powerful metaprogramming capabilities allow for code generation at compile time, enabling developers to create highly customized and optimized code. This capability sets D apart from many other languages, empowering developers to create domain-specific languages (DSLs) or perform complex code transformations during compilation. However, mastering D's metaprogramming features requires a strong understanding of the language's internal workings and a disciplined approach to code organization.
Case Study 1: A compiler development team used D's metaprogramming features to build a highly optimized compiler for a new programming language, significantly reducing compilation time compared to traditional compiler development methods. The use of compile-time code generation enabled the creation of highly optimized compiler internal structures and algorithms. Case Study 2: A graphics rendering engine utilized D's metaprogramming to generate highly optimized shader code at compile time, enhancing the performance of graphics rendering, thereby improving the speed and efficiency of rendering complex graphics.
D's metaprogramming features, such as compile-time function execution (CTFE) and templates, provide immense power but also introduce complexities. Careless use of metaprogramming can lead to cryptic errors and difficult-to-debug code. A structured and well-documented approach is crucial for creating maintainable and robust metaprograms. Understanding the limitations of CTFE and the potential performance implications of complex template instantiations is essential.
Effective use of metaprogramming in D requires a deep understanding of the language's type system and its interaction with the compiler. The ability to generate code tailored to specific situations allows for significant performance improvements and increased code clarity in certain contexts. However, careful planning and modular design are paramount to avoid the pitfalls of excessively complex metaprograms that become unwieldy and difficult to maintain.
Leveraging D's Standard Library: Efficiency and Elegance
D's comprehensive standard library is a treasure trove of well-designed and efficient algorithms and data structures. It provides a solid foundation for building robust and performant applications, minimizing the need for manual implementation of common functionalities. The standard library is not merely a collection of utilities; it represents a carefully curated set of tools that embody best practices in software design and efficiency. However, effectively leveraging the standard library requires understanding its structure and capabilities.
Case Study 1: A data processing pipeline leveraged D's standard library's optimized algorithms for sorting and searching, leading to significant improvements in processing speed and efficiency. The use of these pre-optimized algorithms greatly simplified the development process while maintaining high performance. Case Study 2: A networking application utilized D's standard library's robust networking components for building a scalable and reliable communication framework, ensuring efficient handling of network traffic and robust error handling.
The standard library provides a wealth of high-level abstractions that significantly simplify common programming tasks, such as file I/O, network communication, and string manipulation. This facilitates faster development cycles and reduces the likelihood of introducing errors. Careful selection of appropriate algorithms and data structures from the standard library contributes to the overall performance and maintainability of the software. It is a key element in building efficient and reliable systems.
Familiarity with D's standard library is paramount to writing efficient and idiomatic D code. Understanding the performance characteristics of different algorithms and data structures allows for informed decisions that significantly impact application performance. It significantly simplifies common tasks and promotes code reusability, making it an essential element for any D programmer.
Integrating D with Existing Systems: Interoperability and Practicality
D's interoperability with C and C++ is a key feature that allows for seamless integration with existing codebases. This feature is crucial in migrating legacy systems or integrating D components into larger systems developed in other languages. However, effective integration requires a deep understanding of the interoperability mechanisms and potential challenges.
Case Study 1: A large enterprise migrated a critical component of their legacy system from C++ to D, leveraging D's high performance and improved safety features while seamlessly integrating the updated component with the existing C++ codebase. The use of D's interoperability features allowed for a smooth transition without sacrificing performance or functionality. Case Study 2: A robotics team integrated a D-based control system with a C-based sensor interface, utilizing D's powerful features to process sensor data efficiently while interfacing smoothly with the existing C code.
D’s ability to call C and C++ code directly, and vice versa, makes it a compelling choice for projects needing to leverage existing libraries or legacy code. Understanding the nuances of data marshaling between D and C/C++ is essential for avoiding common errors. This capability expands the possibilities for utilizing D's advantages within pre-existing frameworks.
Effective integration with existing systems often involves careful consideration of memory management and data structures. Differences in memory models and data representations between D and other languages can introduce unexpected behavior if not handled properly. Careful attention to detail and a thorough understanding of the interoperability mechanisms are essential for building robust and reliable hybrid systems. Mastering these techniques unlocks the potential of blending the strengths of D with the realities of existing code bases.
Conclusion
D programming language, while not as widely adopted as some of its contemporaries, possesses a unique blend of power and elegance. This exploration beyond the surface level reveals its capacity for creating high-performance, reliable, and efficient software. From its sophisticated memory management system to its robust concurrency features and powerful metaprogramming capabilities, D offers a compelling alternative for developers seeking higher levels of control and performance. The standard library, coupled with its interoperability with existing C and C++ systems, further enhances its practicality and appeal. By understanding and mastering these key aspects, developers can harness the true potential of D and unlock new levels of efficiency and performance in their applications.