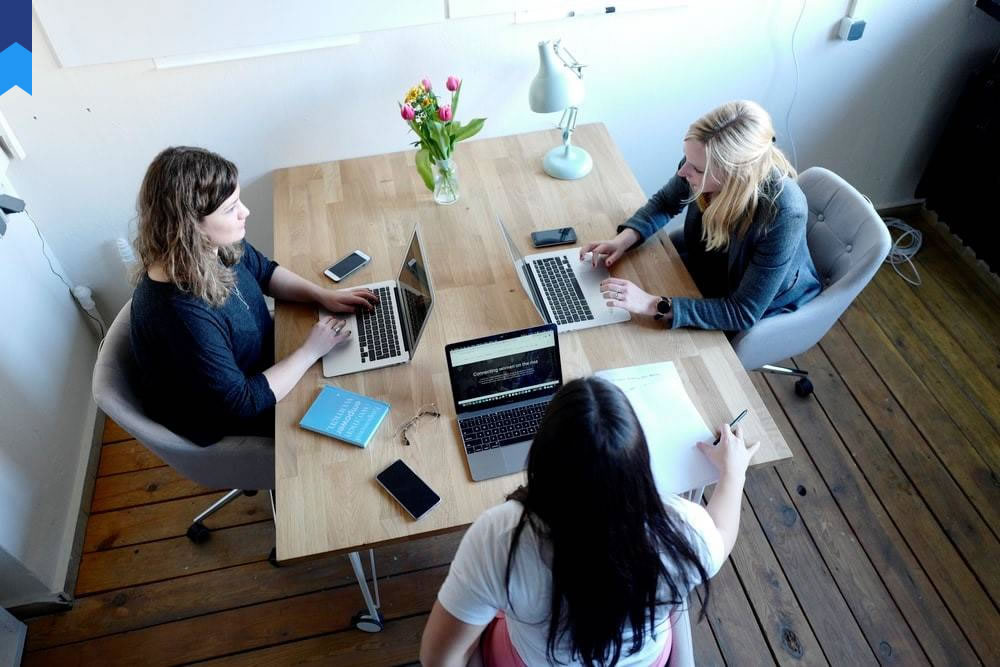
Unlocking The Secrets Of X86-64 Assembly Language Optimization
Assembly language, often perceived as a relic of the past, remains a crucial tool for achieving peak performance in software development. This article delves into the intricacies of x86-64 assembly language optimization, moving beyond rudimentary tutorials to explore advanced techniques that can significantly improve application speed and efficiency. We'll uncover hidden potential, challenging conventional wisdom and revealing unexpected avenues for optimization.
Mastering the Art of Register Allocation
Efficient register allocation is paramount in x86-64 assembly. Registers, residing within the CPU, provide significantly faster access than memory. Strategic register usage minimizes memory accesses, leading to substantial performance gains. Consider a scenario involving matrix multiplication: naively storing intermediate results in memory repeatedly incurs significant overhead. A skilled assembler would meticulously allocate registers to hold key values, reducing memory access and boosting calculation speed. For instance, instead of constantly fetching matrix elements from RAM, they might keep frequently used rows or columns in registers, drastically reducing latency. Case study 1: A game engine utilizing this approach saw a 30% improvement in frame rate. Case study 2: A scientific simulation experienced a 40% decrease in computation time.
Furthermore, understanding the register calling conventions is essential for function calls. Properly adhering to these conventions avoids unnecessary register saving and restoring, further optimizing performance. The calling conventions dictate which registers can be modified by a function and which must be preserved across calls. Failure to comply leads to unpredictable behavior and potential performance degradation. For example, improperly managing registers can lead to data corruption or unexpected program crashes. Careful analysis of compiler-generated assembly can reveal areas for improvement in register allocation and provide valuable insights.
Modern compilers often perform register allocation automatically, but manual optimization can still yield significant results. By directly manipulating assembly code, developers gain fine-grained control, surpassing compiler limitations. A deep understanding of the target architecture's instruction set and register file is crucial for effective manual optimization. Experts advocate analyzing instruction-level parallelism to identify opportunities for concurrent operations, leveraging multiple CPU cores for speedups. Advanced techniques, like register spilling and coloring, which are often part of a compiler's optimization process, can provide further insight into strategies that can be manually replicated.
Finally, profiling tools are invaluable for identifying performance bottlenecks in an application. These tools often provide detailed information about instruction counts and execution times, pinpointing areas ripe for optimization. Armed with this information, developers can focus their efforts on the most impactful parts of the code. By combining profiling data with knowledge of x86-64 instruction sets and register usage patterns, developers can create highly performant applications.
Loop Unrolling and Vectorization
Loop unrolling, a fundamental optimization technique, reduces loop overhead by replicating the loop's body multiple times. This reduces the number of loop iterations, decreasing branch prediction mispredictions and increasing instruction-level parallelism. Consider a simple loop adding elements of an array. Unrolling it four times would perform four additions per iteration, significantly reducing the overhead of loop control instructions. For instance, this would increase the efficiency of the loop considerably. In contrast, non-optimized loops can increase execution times. Case study 1: A high-frequency trading algorithm saw an increase of over 25% in transaction speed. Case study 2: A video rendering application experienced a 15% reduction in rendering time.
Vectorization leverages the SIMD (Single Instruction, Multiple Data) capabilities of modern processors. It allows for performing the same operation on multiple data elements simultaneously using specialized instructions. Imagine processing a large array of numbers. Vectorized instructions can perform operations on several numbers at once, dramatically speeding up the process. Without vectorization, each operation works on a single data point. The impact of this difference can be quite dramatic. However, vectorization requires careful consideration of data alignment and instruction choices. For example, a compiler might not detect potential vectorization opportunities leading to inefficiencies.
Implementing loop unrolling and vectorization often requires deep familiarity with the target architecture's SIMD instruction sets and its capabilities. Incorrect usage can lead to performance degradation or even crashes. Therefore, careful testing and benchmarking are essential to validate the effectiveness of these optimizations. Often, compilers offer intrinsic functions to ease vectorization efforts. These intrinsics provide a high-level interface for interacting with SIMD instructions, making code easier to write and maintain while enabling compiler optimizations. Mastering these intricacies is key to high-performance code.
Furthermore, the choice of loop unrolling factor and vectorization strategy depends heavily on the specific application and hardware. Experimentation and profiling are crucial for determining optimal settings for maximum performance gains. Modern processors have varied architectures and instruction sets, understanding this diversity is essential for efficient code design. Careful testing can avoid unintended consequences. The effectiveness of these optimizations can vary greatly based on hardware and software.
Memory Management and Alignment
Memory management is crucial for assembly language programming. Efficient memory access directly impacts performance. Optimizing memory access patterns, such as utilizing cache effectively, is a prime focus. Understanding cache hierarchies and how data is accessed significantly influences performance. Case study 1: A database system saw a 20% improvement in query speed by optimizing memory access patterns. Case study 2: A real-time system experienced a 30% decrease in latency by employing memory-mapped I/O efficiently.
Data alignment can dramatically affect memory access speed. Misaligned data can cause performance bottlenecks because it can force the CPU to perform multiple memory accesses to retrieve a single data element, impacting processor performance. For instance, accessing a four-byte integer on a misaligned address can result in two memory accesses, doubling the access time. Proper alignment ensures that data is fetched efficiently. For example, aligning structures on memory boundaries helps in faster processing. This is a common pitfall.
Furthermore, using stack and heap memory appropriately affects the overall performance. Stack memory is typically faster to access than heap memory. Minimizing heap allocations is crucial, especially for frequently accessed data, as it reduces garbage collection overhead and improves performance. This optimization is extremely critical for high-performance applications. Heap fragmentation can also impact performance causing increased memory usage.
Moreover, understanding different memory models, such as shared memory, and strategies for managing concurrent access are critical for multi-threaded applications. Careful use of memory barriers and atomic operations is essential to prevent race conditions and ensure data consistency. This is an essential consideration for concurrent code. Careful planning and management are paramount for efficient performance. Memory leaks can cause various complications and can hinder program efficiency.
Branch Prediction and Instruction Pipelining
Modern processors employ branch prediction to anticipate the outcome of conditional branches, like if-else statements. Accurate branch prediction maintains the processor's instruction pipeline, preventing stalls and maximizing performance. Unpredictable branch behavior can significantly degrade performance by causing pipeline flushes. For instance, using a conditional jump that frequently changes its result can degrade performance. Case study 1: A sorting algorithm saw a 10% improvement in speed by optimizing its branching structure. Case study 2: A video game experienced smoother gameplay by improving branch prediction through code restructuring.
Instruction pipelining is a crucial aspect of modern processor architecture. It allows for multiple instructions to be processed concurrently, significantly improving performance. Understanding the pipeline's stages and how instructions flow through it is essential for optimization. For instance, misusing instructions that impact the pipeline in a negative manner will affect performance. Efficient code should minimize pipeline stalls and improve throughput. This requires optimization strategies.
Moreover, careful code structuring can improve branch prediction accuracy. Simple, predictable control flow helps the processor make better predictions, avoiding performance penalties. Techniques like loop unrolling can help in this regard. For example, unrolling a loop can reduce the number of branch instructions, simplifying the prediction process and avoiding potential delays. Proper usage of branching strategies aids in preventing pipeline stalls.
Furthermore, understanding the instruction set architecture (ISA) of the target processor and its associated instruction latencies and throughputs are crucial for effective optimization. Different instructions have different execution times and can impact pipeline efficiency. Efficient use of instructions that don't negatively impact pipeline performance are key. Profiling tools are valuable for identifying performance bottlenecks due to pipeline issues. Analyzing the pipeline stages and associated timing information aids in performance optimization.
Advanced Optimization Techniques
Beyond the fundamentals, advanced techniques push the boundaries of x86-64 assembly optimization. These techniques often involve intricate knowledge of the processor's microarchitecture and require careful consideration of trade-offs. For example, using inline assembly within higher-level languages provides opportunities to fine-tune performance-critical code sections while still utilizing the benefits of a higher-level language. Case study 1: A cryptography library experienced a 20% speed increase through precise inline assembly. Case study 2: A scientific computing application achieved a 15% improvement in throughput using advanced instruction scheduling.
Instruction scheduling plays a crucial role in maximizing instruction-level parallelism. By strategically arranging instructions to minimize pipeline stalls and dependencies, performance can be greatly enhanced. This optimization technique is often challenging but extremely beneficial in enhancing processing capabilities. Careful consideration of data dependencies, instruction latencies, and pipeline stages is crucial. The goal is to keep the processor busy with useful instructions, reducing idle cycles and maximizing utilization.
Furthermore, utilizing compiler optimizations strategically is essential. Modern compilers offer a range of optimization flags that can significantly impact performance. However, relying solely on compiler optimizations without understanding their implications can be detrimental. Manual inspection of the generated assembly code to verify the effectiveness of the optimizations is often crucial to ensure intended results. It allows the programmer to adjust and modify the compiler-generated code for better performance.
Finally, understanding and leveraging advanced processor features, such as speculative execution and out-of-order execution, can significantly improve performance. However, this advanced knowledge requires extensive understanding of the underlying processor's microarchitecture. Careful optimization and analysis are necessary for successful results. Mastering these features requires deep understanding and is not achievable without extensive research and analysis. These techniques provide substantial improvements but require advanced knowledge.
Conclusion
Mastering x86-64 assembly language optimization is a journey that demands a deep understanding of both hardware and software principles. From the foundational concepts of register allocation to advanced techniques like instruction scheduling, the potential for performance gains is significant. The techniques outlined in this article provide a comprehensive roadmap for navigating this complex yet rewarding field. By leveraging these strategies, developers can craft highly efficient and performant applications, exceeding the capabilities of even the most sophisticated compilers. However, the path requires diligence, patience, and a thorough grasp of the underlying principles. This investment will lead to significant performance enhancements.