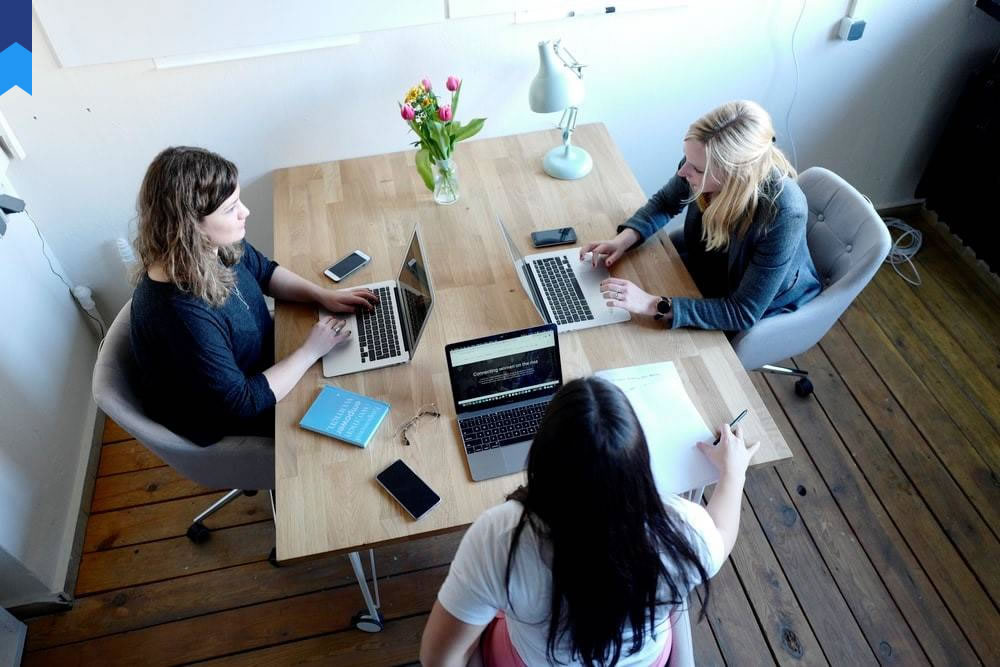
What Async Await Can Teach Us About Dart's Concurrency
Dart's concurrency model is powerful, yet mastering it requires understanding its nuances. This article delves into the practical applications of asynchronous programming with a focus on the often-misunderstood `async` and `await` keywords, showing how they simplify complex asynchronous operations and enable efficient, responsive Dart applications. We'll explore real-world scenarios, demonstrating how proper utilization of these keywords can significantly improve application performance and maintainability.
Understanding Dart's Event Loop and Futures
Dart employs a single-threaded event loop architecture. This means only one task executes at a time. However, Dart excels at handling I/O-bound tasks (like network requests or file operations) asynchronously without blocking the main thread. This is achieved using Futures, which represent the eventual result of an asynchronous operation. The `Future` object itself doesn't contain the result immediately; instead, it signals completion once the result is available. Without `async` and `await`, working with Futures involves using `.then()` chains, leading to complex, nested structures that quickly become unreadable.
Case Study 1: Imagine fetching data from multiple APIs. Using `.then()` for each API call results in a deeply nested structure. This makes debugging and understanding the code flow extremely difficult. `async`/`await` transforms this into a linear, synchronous-looking structure, enhancing readability significantly.
Case Study 2: Consider a scenario where you need to process a large file. Blocking the main thread while reading the entire file would freeze the application. Asynchronous file reading, combined with `async`/`await`, allows you to process the file in chunks, keeping the UI responsive. This improves user experience and prevents application crashes due to resource exhaustion. This strategy is particularly crucial in mobile application development where limited resources necessitate efficient concurrency management.
The core principle here is to offload lengthy operations to background threads, allowing the main thread to remain responsive. The event loop efficiently manages these asynchronous tasks, switching between them as they complete, preventing blocking and maximizing resource utilization. This asynchronous model, coupled with the elegance of `async` and `await`, is central to building high-performance Dart applications.
A common pitfall is neglecting error handling. Without proper error handling within asynchronous operations, exceptions could go uncaught, leading to unexpected application behavior. Always ensure your `async` functions have appropriate `try-catch` blocks to handle potential exceptions and gracefully recover from errors. Efficient error handling directly contributes to application stability and reliability.
Simplifying Asynchronous Operations with async and await
The `async` keyword transforms a function into an asynchronous function. This allows the use of the `await` keyword within the function. `await` pauses execution of the function until the `Future` it's waiting on completes, returning its result. This dramatically simplifies asynchronous code, allowing developers to write code that reads like synchronous code, without the complexities of nested callbacks.
Case Study 1: Consider a scenario where a user needs to log in. Fetching user data involves a network request, which is asynchronous. With `async`/`await`, you can write the login logic as if it were synchronous, significantly improving code readability and maintainability. This approach also simplifies testing as the code resembles synchronous code.
Case Study 2: In a real-time application, handling multiple concurrent data streams often involves complex asynchronous operations. `async`/`await` significantly simplifies the management of these streams, making code easier to reason about and reducing the risk of errors. This streamlined approach results in more efficient development and easier debugging.
The power of `async`/`await` lies in its ability to make asynchronous code look and behave like synchronous code, thereby increasing the readability and maintainability of your applications. This makes it easier for developers to understand and debug their code, leading to faster development cycles and higher quality applications.
It is important to note that while `await` pauses the execution of the *current* function, it doesn't block the entire event loop. The event loop continues processing other events, ensuring application responsiveness.
Streamlining Data Processing with async/await and Streams
Streams in Dart represent a sequence of asynchronous events. Combined with `async`/`await`, Streams provide a powerful mechanism for processing asynchronous data efficiently. The `await for` loop allows you to iterate over a stream, pausing execution until the next data element becomes available. This simplifies the handling of large datasets or continuous data streams.
Case Study 1: Suppose you're building a chat application. Incoming messages arrive as a stream of events. Using `await for`, you can easily process each message as it comes in, updating the UI in real time. This ensures the user interface remains responsive and provides a seamless user experience.
Case Study 2: Imagine processing a large log file. Instead of loading the entire file into memory, you can treat the log file as a stream, processing lines individually. This approach drastically reduces memory consumption, making it possible to process incredibly large log files without causing application crashes.
By effectively utilizing Streams with `async`/`await`, you can create highly efficient and responsive applications capable of handling significant data volumes. This ensures efficient resource utilization and a smooth user experience, especially crucial in applications handling real-time data.
The combination of Streams and `async`/`await` provides a powerful and elegant solution for managing asynchronous data. It significantly improves code readability and maintainability compared to traditional callback-based approaches. Adopting this approach leads to more robust and scalable applications.
Advanced Techniques: Isolates and Parallel Processing
While Dart’s event loop handles asynchronous operations efficiently, for computationally intensive tasks, leveraging isolates can significantly improve performance. Isolates provide true parallelism, allowing you to run code in separate threads, independent of the main event loop. This is especially beneficial for tasks that are CPU-bound rather than I/O-bound.
Case Study 1: Imagine performing complex image processing or data analysis. Using isolates, you can delegate these tasks to separate threads, leaving the main thread free to handle user interactions. This results in a highly responsive application, even under heavy computational loads.
Case Study 2: In a machine learning application, training a model can be a computationally expensive task. Offloading this to a separate isolate prevents freezing the UI while the model trains, significantly improving user experience.
Communicating between isolates involves using `SendPort` and `ReceivePort`, which allows data exchange between the isolates. This is generally slower than communication within the same thread, so it’s important to consider the overhead when choosing this approach. Prioritize CPU-bound tasks for isolation to maximize its benefits.
The effective use of isolates, in combination with `async`/`await` for managing communication between the main isolate and worker isolates, creates a powerful concurrency model for building highly performant Dart applications. Strategic use of isolates prevents blocking the main thread, enabling responsiveness even under significant computational load.
Best Practices and Common Pitfalls
While `async`/`await` simplifies asynchronous code, several best practices ensure optimal performance and maintainability. Always handle exceptions gracefully using `try-catch` blocks to prevent unexpected application behavior. Avoid excessive nesting of `async` functions; keep the structure clear and concise for readability. Overuse of `await` can hinder performance in some cases; if waiting for many Futures, consider using `Future.wait` for efficient batch processing.
Case Study 1: Consider an application that makes many concurrent API calls. Using `Future.wait` will result in increased efficiency compared to awaiting each Future individually, reducing unnecessary context switching.
Case Study 2: In a complex application, improper exception handling can lead to cryptic error messages and unexpected application crashes. Employing robust error handling across all asynchronous operations prevents this.
Choosing between using futures directly or utilizing `async`/`await` depends on the context. For simpler scenarios, futures might suffice, but for more complex operations, `async`/`await` enhances readability and maintainability significantly. Understanding the trade-offs and following best practices leads to more robust and efficient Dart applications.
Remember, the goal is to write clean, maintainable code that is easy to understand and debug. By adhering to these best practices and understanding the underlying mechanisms of Dart's concurrency model, developers can create highly responsive and efficient applications.
Conclusion
Mastering Dart's concurrency model is essential for creating high-performance, responsive applications. The `async` and `await` keywords are powerful tools that significantly simplify asynchronous programming, enabling developers to write clean, readable code. By understanding the nuances of Dart's event loop, Futures, Streams, and Isolates, and by following best practices, developers can leverage the full potential of Dart's concurrency model to build robust and scalable applications. From handling network requests to processing large datasets, `async`/`await` provides a clear and efficient path toward building sophisticated, responsive applications.
This article has explored the various aspects of using `async`/`await` in Dart, demonstrating its power in simplifying complex asynchronous operations. Proper implementation leads to more maintainable, efficient, and reliable applications. By adopting these techniques, developers can significantly improve their Dart development workflow and create higher-quality software.