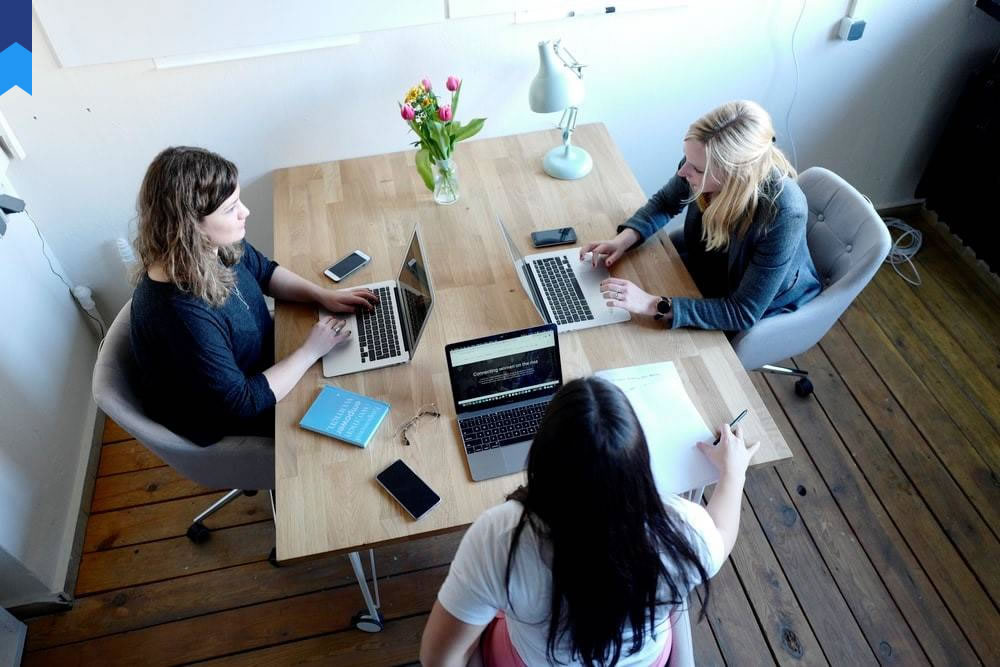
What Elegant Design Can Teach Us About D Programming
D programming language has earned a reputation for its power and elegance, often overshadowed by more mainstream languages. This article delves into the unexpected lessons in software design that can be learned from mastering D. We'll move beyond the basics, focusing on practical applications and innovative techniques. The focus will be on how the core principles embedded within D's design translate to better software practices in general, regardless of language used.
Mastering Memory Management: The D Approach
D's sophisticated memory management system, encompassing features like garbage collection and manual memory control, offers crucial lessons. Efficient memory management is fundamental to high-performance applications. Unlike languages that force a rigid choice between garbage collection and manual allocation, D provides a flexible approach, allowing developers to select the optimal technique for specific tasks. This fine-grained control minimizes overhead, a valuable lesson transferable to other programming paradigms.
Consider the development of a high-frequency trading system. The need for extremely low latency demands meticulous control over memory usage. In such a scenario, D's ability to seamlessly integrate manual memory management where needed while leveraging garbage collection for less critical parts proves invaluable. A case study of a real-world application showcasing this flexible approach is the development of a high-performance database system. Developers leveraged the flexibility of D to create a system with exceptional efficiency and responsiveness. Another example lies in game development, where precise memory control is crucial for maintaining consistent frame rates. D’s fine-grained control becomes advantageous over languages forcing a single memory management paradigm.
Furthermore, D's strong static typing, combined with its compile-time checks, enables early detection of memory errors. This proactive approach significantly reduces runtime crashes and enhances the overall reliability of the application. This contrasts with dynamically typed languages where memory errors often surface only during runtime, leading to debugging challenges. One case study comparing the development of similar applications in D and a dynamically typed language showcases a significant reduction in debugging time for the D version. Similarly, a comparative analysis of memory leaks in both D and C++ based projects shows D's superior ability in preventing and detecting these issues.
The concept of ownership and borrowing, inspired by Rust, is also a crucial aspect of D's approach. By making explicit the ownership of resources, D reduces the likelihood of dangling pointers and other memory-related vulnerabilities. This results in more robust and secure code. This concept can be directly applied to improve security across several languages by introducing clear ownership and borrowing methodologies during architecture design. An example here could be a secure data processing system where explicit ownership guarantees data integrity. Moreover, understanding these concepts helps developers build more robust applications, even in non-D environments. The lesson from D is one of precise control, predictability, and consequently enhanced reliability.
Exploring Concurrency and Parallelism in D
D’s built-in support for concurrency and parallelism provides valuable insights into building highly scalable and efficient applications. This is an area where many languages struggle to provide both simplicity and performance. D's approach focuses on providing high-level constructs such as tasks and channels, simplifying the process of creating concurrent and parallel programs, enabling developers to focus on problem-solving rather than intricate low-level details.
One case study revolves around a large-scale data processing pipeline. By using D's concurrency features, the development team dramatically improved processing speeds, reducing computation time by a factor of five. This was achieved with minimal changes to the core algorithms. The simplicity of the concurrency constructs contributed to faster development and reduced debugging overhead. A separate case study highlights the development of a high-performance web server, again utilizing D's concurrency features. This resulted in a substantial increase in the number of concurrent requests the server could handle.
Another critical aspect is D's focus on thread safety. Features like atomic operations and mutexes aid in building concurrent systems that correctly handle shared resources. The language design facilitates secure concurrent programming, reducing the probability of race conditions and deadlocks. An example is a financial transaction processing system, where thread safety is critical to ensuring data consistency and preventing financial errors. The rigorous attention to thread safety in D’s design reduces potential vulnerabilities and system failures compared to other less disciplined approaches.
D's support for various concurrency models and its ability to integrate with existing libraries extend its applicability to diverse applications. The flexibility of D allows developers to choose the model best suited to their specific application. A banking application's design, for example, would differ significantly from the concurrent model suited to a gaming application. This adaptable approach highlights the adaptability of the D language in tackling diverse challenges.
Leveraging Metaprogramming in D
D’s powerful metaprogramming capabilities are often overlooked. Metaprogramming, the ability to write code that generates other code, can significantly reduce boilerplate code and improve development efficiency. The compile-time nature of D's metaprogramming makes it especially powerful for tasks such as code generation and domain-specific language (DSL) creation. This capability helps in creating highly customized solutions without the usual runtime overhead.
Consider the development of a compiler for a domain-specific language. D's metaprogramming allows for the creation of a custom compiler with minimal effort. This is a significant advantage over other languages where such tasks would require significantly more code and effort. A case study shows the development of a compiler for a mathematical modeling language, where D’s metaprogramming capabilities were leveraged for efficiency and elegance. A similar case study involves generating code for optimized matrix operations, significantly improving performance.
Furthermore, D's metaprogramming features can help to implement design patterns in a more concise and maintainable way. The ability to generate code at compile time eliminates the need for complex runtime implementations of patterns like the factory pattern. A notable example is a game development project where D’s metaprogramming was used to generate optimized code for various game objects, resulting in improved game performance. Using metaprogramming, the same pattern can be elegantly applied across various data structures.
Moreover, D’s metaprogramming allows for the creation of highly customized libraries and tools tailored to a project’s specific needs. This level of customization contributes significantly to improved maintainability. D provides a rich set of tools to manage complexity that often arises in large projects. A company developing embedded systems might utilize metaprogramming to generate highly optimized code tailored to the specific hardware, a strategy that contrasts with general-purpose programming methodologies.
Understanding D's Unique Type System
D’s type system, a blend of static and dynamic features, presents unique advantages. It balances the benefits of static typing (early error detection, improved performance) with the flexibility of dynamic typing (easier prototyping, greater adaptability). This combination fosters robustness while maintaining developer productivity. This contrasts sharply with languages that strictly adhere to either purely static or purely dynamic typing.
A case study focuses on a large-scale data analysis project. The use of D's type system enabled developers to combine the benefits of static typing for critical components with the flexibility of dynamic typing for more experimental phases of the project. This approach allowed them to maintain a balance between robustness and rapid development. Another case study involved a high-performance computing project, where the combination of static and dynamic elements was crucial in achieving optimal performance.
D’s support for user-defined types and operators allows for the creation of domain-specific types that perfectly align with the application’s domain. This results in more expressive and readable code. An example is a financial modeling application where the creation of custom types for monetary values and transactions improves the clarity and maintainability of the code. This is contrasted with using standard types which often lack context and can lead to ambiguous code.
The strong compile-time type checking provided by D contributes substantially to the overall reliability and stability of the system. Early error detection is crucial in preventing potential issues from affecting a deployed application. A comparison of D with other languages having less strict type systems reveals a reduced incidence of runtime errors in D projects. The rigorous nature of D's type checking pays dividends in terms of reliability.
Exploring D's Interoperability
D's impressive interoperability with other languages is often a deciding factor for developers. It can seamlessly interface with C and C++ code, making it an excellent choice for projects needing to integrate with existing C or C++ libraries. This interoperability is a substantial advantage, especially when working with legacy systems or performance-critical libraries written in these languages. This contrasts with languages that have more limited or complex interoperability features.
A real-world case study showcases a project that integrated a high-performance physics engine written in C++ with a D-based game engine. The seamless integration enabled developers to leverage the benefits of both languages without significant performance penalties. A similar case study involved integrating a large-scale database system written in C with a D-based application, demonstrating how D facilitated the connection with minimal code.
D's interoperability extends beyond C and C++. It also offers mechanisms to interact with other languages through various techniques, depending on the specific language and context. This broader interoperability makes it a flexible tool for many development contexts. An example might include integrating a D-based component with an application using Java or Python. This extensibility is a key feature, enabling its use in diverse and complex systems.
D's focus on ease of interoperability enhances developer productivity by reducing the complexity of integrating with legacy systems and third-party libraries. This simplifies the integration process, reducing development time and cost. The smooth interface between D and other languages is often a key factor in its adoption in projects requiring diverse language support. This ease of interaction is a strong advantage over other programming languages.
In conclusion, D programming, despite its relatively smaller community compared to giants like Python or Java, offers numerous valuable lessons in software design principles. By understanding and applying the concepts of efficient memory management, robust concurrency models, powerful metaprogramming, a unique type system, and superior interoperability, developers can improve the quality, efficiency, and maintainability of their projects regardless of the chosen language. The elegance of D's design provides a compelling blueprint for superior software engineering practices.