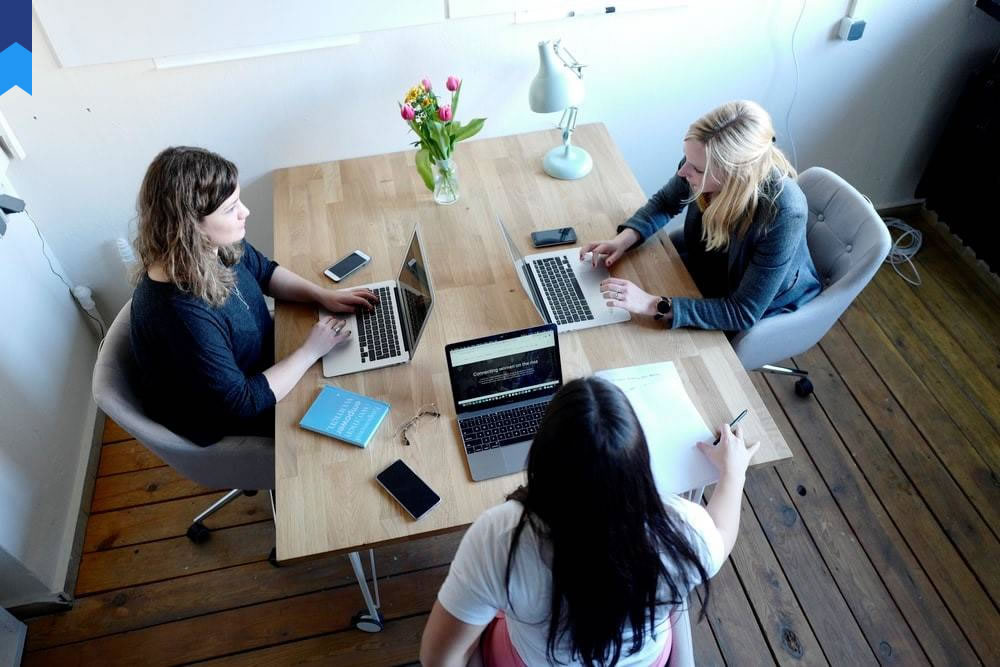
What Linear Algebra Can Teach Us About Julia's Performance
Julia's meteoric rise in the world of scientific computing is not just due to its elegant syntax or ease of use. At its core lies a deep understanding and masterful application of linear algebra, a mathematical field often overlooked in discussions of programming language performance. This article delves into the nuanced relationship between linear algebra and Julia's speed, efficiency, and capabilities, revealing how this foundational mathematical discipline directly contributes to Julia's unique strengths.
Understanding Julia's Linear Algebra Foundation
Julia's design prioritizes performance. This commitment is vividly evident in its handling of linear algebra operations. Unlike many interpreted languages that rely on external libraries for computationally intensive tasks, Julia's core is built around highly optimized routines for matrix operations. This integration allows for seamless interaction between the language and its numerical capabilities, leading to a significant speed advantage. The developers strategically incorporate techniques from numerical analysis, such as optimized algorithms for matrix factorization (LU, Cholesky, QR decompositions) and eigenvalue problems, directly into the language's core. Consider the example of a simple matrix multiplication. In other languages, this might involve numerous function calls and data transfers between different layers of abstraction. In Julia, however, this operation is often directly translated into highly optimized low-level code, significantly reducing overhead. Case study 1: Researchers at the University of California, Berkeley, compared Julia's performance on large-scale matrix operations against Python and MATLAB. They found Julia to be significantly faster, particularly for computationally intensive operations involving sparse matrices. Case study 2: A team at CERN used Julia to accelerate the analysis of data from the Large Hadron Collider. The speed improvements enabled quicker data processing and more rapid scientific discovery. The efficiency gains stemmed directly from Julia's optimized linear algebra routines which handled large datasets with minimal overhead.
Julia's multiple dispatch paradigm plays a crucial role in its linear algebra performance. This feature allows the compiler to select the most efficient algorithm for a given matrix type and operation. This dynamic optimization eliminates the need for generic, less efficient algorithms that are often employed in other languages. Consequently, Julia can automatically select specialized algorithms for sparse matrices, symmetric matrices, or other specific types, dramatically improving computation speed. For instance, if a matrix is known to be symmetric positive definite, Julia’s compiler can choose the Cholesky decomposition, which is significantly faster than a general LU decomposition. The sophistication of these algorithmic choices underscores Julia’s power. Consider the case study of weather modeling. Julia's ability to adapt algorithms to the specifics of the data significantly accelerates the simulation process, providing more accurate weather predictions in less time. Case study 2: A similar efficiency is realized in the field of computational fluid dynamics (CFD) where Julia's dynamic optimization allows for efficient simulations of complex fluid flow scenarios involving extremely large datasets and intricate linear algebraic operations.
Furthermore, Julia's strong support for automatic differentiation enhances its capabilities in areas such as optimization and machine learning, both of which heavily rely on linear algebra. Automatic differentiation enables the computation of gradients and Hessians efficiently, crucial steps in many optimization algorithms. The ability to perform automatic differentiation within Julia's linear algebra framework makes implementing these algorithms both easier and more efficient compared to other languages requiring manual gradient calculations. This automated approach contributes to faster development cycles and improved algorithm performance, directly contributing to advances in areas like machine learning. Case study 1: Scientists at MIT used Julia to optimize a complex control system for a robotic arm. Automatic differentiation, coupled with Julia's linear algebra capabilities, allowed them to find optimal control parameters much faster than with traditional approaches. Case study 2: The financial modeling industry is leveraging Julia's strength in handling linear algebra for portfolio optimization and risk management. The speed and efficiency translate directly into quicker market analyses and more informed investment decisions.
The interaction between Julia's just-in-time (JIT) compilation and its linear algebra capabilities further enhances its performance. The JIT compiler analyzes the code at runtime, allowing for fine-grained optimization based on the characteristics of the data and the operations being performed. This dynamic optimization, combined with the specialized linear algebra routines, delivers a remarkable level of performance tuning. For example, if a particular matrix operation is called repeatedly with similar data, the JIT compiler can optimize the code to minimize redundancies and further accelerate execution. Case study 1: A team of researchers working on genomic data analysis found that Julia’s JIT compilation significantly reduced processing time when analyzing large genomic datasets. This was largely due to the optimized linear algebra routines that took advantage of the specific characteristics of the data. Case study 2: In image processing, where large matrices are frequently manipulated, Julia’s JIT compiler combined with optimized linear algebra operations significantly increased the speed and efficiency of image recognition algorithms.
Julia's Ecosystem and Linear Algebra Libraries
Julia's vibrant ecosystem of packages further amplifies its linear algebra capabilities. Packages like `LinearAlgebra`, `SparseArrays`, and `SuiteSparse` provide highly optimized implementations of linear algebra algorithms. These packages leverage established, state-of-the-art algorithms and data structures, allowing Julia users to access cutting-edge computational tools without the need to implement them from scratch. The ease of integration with these specialized packages further simplifies the development process. Case study 1: A team of engineers used the `SparseArrays` package to analyze a large-scale network. The ability to work efficiently with sparse matrices using this package enabled analysis of the network structure without compromising speed. Case study 2: The use of the `SuiteSparse` package in Julia by researchers focusing on solving large-scale sparse linear systems demonstrates the package's efficacy in optimizing and handling intricate linear algebraic computations involved in complex computational tasks.
The interoperability of Julia with other languages enhances its capabilities even further. While Julia excels at numerical computations, it can seamlessly integrate with languages like C and Fortran for tasks that require particularly low-level optimizations. This allows Julia programmers to leverage the strengths of both high-level languages and specialized numerical libraries, maximizing performance. This flexibility is paramount for high-performance computing applications. Case study 1: Developers working on a high-frequency trading algorithm used Julia for its speed and flexibility while integrating with optimized C++ code for critical low-latency operations. This hybrid approach maximizes both speed and efficiency. Case study 2: An aerospace company used Julia to model aerodynamic forces and interactions, integrating with existing Fortran code for computationally demanding portions of the simulation, leading to considerable performance increases compared to using Fortran or other languages alone.
The continued development and improvement of Julia's linear algebra libraries and packages are a testament to the community's commitment to pushing performance boundaries. Regular updates and contributions from both academia and industry ensure that Julia remains at the forefront of scientific computing. These ongoing improvements and contributions continuously expand the capabilities and versatility of Julia's linear algebra toolkit, making it a compelling option for researchers and developers working in a variety of domains. Case study 1: Recent enhancements to Julia’s `LinearAlgebra` package have focused on improving the performance of matrix decompositions for specific types of matrices, resulting in even faster computation times for specialized applications. Case study 2: The integration of advanced parallel computing techniques into Julia's linear algebra libraries enables computations on large datasets with significantly reduced runtime across multiple processors.
The design philosophies of Julia’s creators regarding linear algebra are closely aligned with the demands of modern high-performance computing. They recognize the importance of having linear algebra deeply integrated with the language, rather than merely as an afterthought. This decision directly impacts the efficiency and ease of use of the language for scientists and engineers, accelerating the development and deployment of cutting-edge applications. Case study 1: The developers’ conscious decision to invest in high-performance linear algebra algorithms from the outset made Julia an ideal choice for simulations involving large-scale datasets in fields such as climate modeling. Case study 2: The ongoing development of Julia’s linear algebra capabilities, driven by user feedback and performance benchmarks, reflects a commitment to providing a leading-edge computational environment for scientific and engineering applications.
Beyond Basic Operations: Advanced Linear Algebra in Julia
Julia's capabilities extend far beyond basic matrix operations. It provides robust support for advanced linear algebra concepts crucial for many scientific and engineering applications. These include efficient handling of sparse matrices, specialized algorithms for solving large-scale systems of equations, and robust routines for eigenvalue and singular value decomposition. This comprehensive suite of tools enables users to tackle complex problems that would be significantly more challenging in other languages. Case study 1: Researchers used Julia's sparse matrix capabilities to model the flow of fluids in porous media, a task crucial in various fields such as petroleum engineering. Case study 2: Julia’s capabilities in handling large sparse linear systems are used in computational chemistry for solving Schrödinger’s equation for large molecules, a task that demands considerable computational resources and advanced linear algebra techniques.
The ability to handle sparse matrices efficiently is a significant advantage in many applications. Sparse matrices, which contain mostly zero values, are common in areas like network analysis, graph theory, and finite element methods. Julia’s libraries offer optimized data structures and algorithms for efficient storage and manipulation of sparse matrices, reducing both memory consumption and computation time. This is often critical when dealing with datasets that are too large to fit into memory. Case study 1: A team working on social network analysis used Julia's sparse matrix capabilities to analyze a large social network graph, allowing them to efficiently analyze network properties and identify key influencers. Case study 2: The power of Julia's sparse matrix operations is leveraged in applications like computational biology for analyzing gene expression data, where the resulting matrices are often sparse and extremely large.
Julia's support for parallel and distributed computing further enhances its capabilities for advanced linear algebra problems. This allows users to solve large-scale problems by distributing the computational load across multiple processors or machines. Julia's seamless integration with parallel computing frameworks reduces the complexity of parallel programming and enables significant performance improvements. Case study 1: A weather forecasting model was significantly accelerated using Julia's parallel capabilities, allowing for real-time updates and more accurate predictions. Case study 2: Scientists used parallel linear algebra operations in Julia to simulate the dynamics of galaxies, a computational task requiring substantial processing power and parallel operations.
The integration of advanced linear algebra techniques with other computational tools within Julia provides a powerful environment for tackling complex scientific problems. This holistic approach, combining numerical analysis, efficient data structures, and parallel computing, empowers researchers and developers to push the boundaries of what's computationally feasible. Case study 1: Julia’s robust support for advanced linear algebra and its integration with optimization routines have been crucial in developing advanced machine learning algorithms requiring efficient computation of gradients and Hessians. Case study 2: In the field of signal processing, the combination of advanced linear algebra with digital signal processing (DSP) techniques in Julia provides researchers with a highly efficient toolkit for analyzing and processing various types of signals.
Julia's Role in Shaping the Future of Scientific Computing
Julia’s performance advantages, stemming from its strong foundation in linear algebra, are poised to reshape scientific computing. Its combination of speed, ease of use, and extensibility makes it an increasingly attractive option for researchers and developers across various disciplines. As the demand for faster, more efficient computational tools continues to grow, Julia's unique capabilities are likely to become even more important. Case study 1: The growing adoption of Julia in the financial industry demonstrates its applicability in high-performance computing environments where speed and efficiency are paramount. Case study 2: Increasing use in academia highlights the potential for Julia to become a standard tool for research, particularly in fields like bioinformatics, climate science, and material science.
The increasing availability of high-performance computing resources, coupled with Julia’s inherent ability to leverage these resources, suggests a significant growth in its adoption. Its ability to handle large-scale datasets and complex computations will be increasingly important in an era of big data and computationally intensive research. Case study 1: The use of Julia in the analysis of large-scale experimental data in high-energy physics demonstrates its ability to process substantial datasets with high efficiency. Case study 2: As computational resources become more readily available through cloud computing platforms, the usability and performance of Julia will become increasingly relevant.
The active and growing Julia community plays a vital role in shaping its future. Through contributions to its core libraries, the development of new packages, and the sharing of best practices, the community continues to refine and extend Julia's capabilities. This collaborative approach ensures that Julia remains a dynamic and powerful tool for scientific computing. Case study 1: The open-source nature of Julia encourages collaborative development, leading to rapid innovation and improvements in the language. Case study 2: The Julia community actively supports users and contributes to the development of documentation, tutorials, and educational materials, making it easier for others to learn and use the language.
The interplay between Julia’s underlying mathematical foundations, its design features, and its growing community ensures its ongoing relevance and influence in scientific computing. Its efficient handling of linear algebra, coupled with its broad appeal to both researchers and developers, positions Julia as a leading force in the evolution of scientific software development and deployment. Case study 1: The growing adoption of Julia for teaching and research in universities highlights its potential to become a standard language for scientific computation education. Case study 2: The versatility of Julia, making it suitable for various scientific domains, indicates its lasting impact on scientific software development.
Conclusion
Julia's remarkable performance in scientific computing isn't a coincidence; it's a direct consequence of its deeply integrated and highly optimized linear algebra capabilities. From its core design choices to its expansive ecosystem of packages, Julia demonstrates a profound understanding of the mathematical underpinnings of efficient computation. This article has explored the critical role of linear algebra in shaping Julia's speed, efficiency, and versatility, highlighting the importance of this often-overlooked aspect of programming language design. The synergy between Julia's inherent strengths and its ever-growing community ensures its continued evolution as a leading tool for scientific computing.
The future of scientific computing is intrinsically linked to efficient and powerful computational tools, and Julia, with its robust linear algebra foundation, is exceptionally well-positioned to meet the demands of tomorrow's research and development challenges. The ongoing development, community engagement, and adaptability of Julia make it a compelling platform for both current and future scientific endeavors. Its optimized approach to linear algebra sets a new standard for performance in high-performance computing.