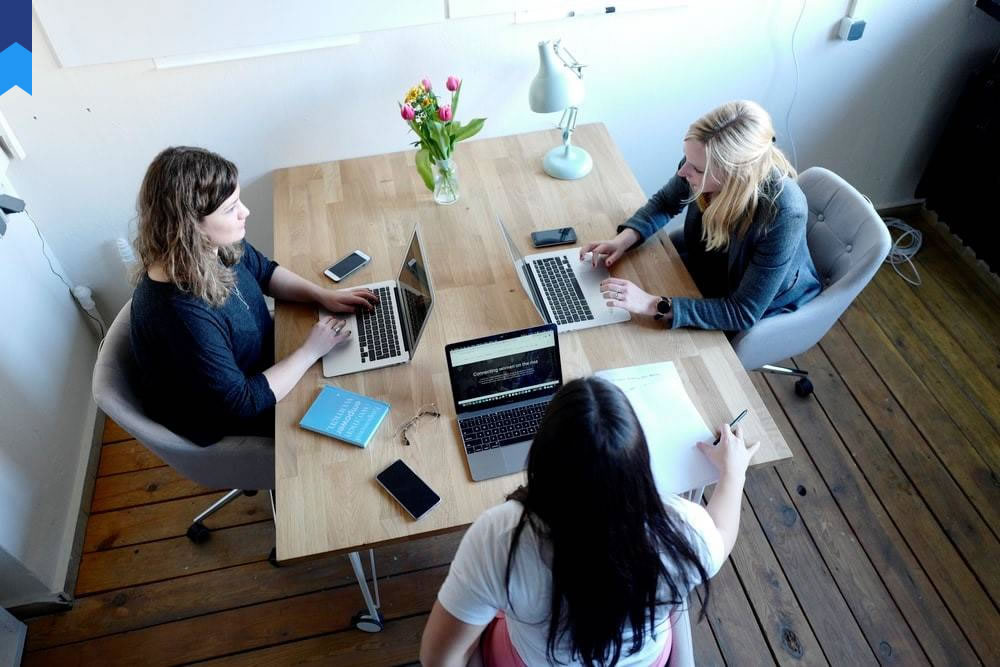
What Rust Experts Don't Tell You About Memory Management
Rust's reputation as a memory-safe language often overshadows the nuances of its memory management system. While the borrow checker prevents many common errors, understanding its intricacies is crucial for writing efficient and robust code. This article delves into those often-overlooked aspects, revealing techniques that seasoned Rustaceans utilize for optimal performance and memory utilization.
Understanding Ownership and Borrowing: Beyond the Basics
Rust's ownership system, while powerful, requires a deep understanding beyond the surface level. The rules surrounding ownership, borrowing, and lifetimes often present challenges even for experienced developers. For instance, the subtle differences between mutable and immutable borrows, along with the complexities of nested borrowing scenarios, can lead to unexpected compile-time errors. Consider the common pitfall of attempting to mutate a value through multiple mutable borrows simultaneously – this directly violates Rust's ownership rules, resulting in a clear and concise error message from the compiler. However, the nuances of lifetime annotations, especially in complex data structures or closures, can be challenging to grasp. Case study: A project attempting to implement a thread-safe data structure might encounter subtle lifetime issues that manifest as runtime panics if lifetimes are not carefully managed. The solution lies in understanding how to explicitly manage lifetimes using `'static` or other lifetime annotations. Another example: When dealing with external libraries, understanding how the library's lifetime annotations interact with your code is critical to avoid unexpected behavior. Efficiently managing lifetimes in these situations often requires careful analysis of the library's documentation and source code.
Furthermore, mastering the art of working with smart pointers is essential. While `Rc
Advanced techniques such as interior mutability allow bypassing some of the restrictions imposed by the borrow checker while maintaining memory safety. Types like `RefCell
Finally, understanding the interaction between ownership, borrowing and generics is crucial for building reusable and flexible code. The compiler's ability to enforce borrowing rules across generic types adds another layer of complexity that demands careful consideration. Improper handling can lead to cryptic compilation errors. Case Study: A generic data structure attempting to implement a borrow checker-friendly linked list encountered difficulties in correctly managing lifetime annotations across different generic types. Proper understanding of generic lifetimes and careful use of lifetime annotations solved the compile-time errors. Another example: A generic algorithm meant to process data structures with varying ownership semantics struggled with lifetime annotation intricacies, resulting in frustrating compile-time errors. Careful structuring of the generic constraints and suitable lifetime annotations solved the issue.
Memory Allocation and Deallocation: Beyond `Box` and `Vec`
While `Box
Utilizing stack allocation whenever possible avoids the overhead associated with heap allocation, leading to improved performance, especially in applications with stringent real-time constraints. Case Study: An embedded system utilizing stack allocation where appropriate minimized memory footprint and improved responsiveness. Another example: A real-time audio processing application employed stack allocation to ensure deterministic execution and low latency. Careful consideration of the trade-offs between stack and heap allocation is essential.
Understanding memory alignment and its impact on performance is often overlooked. Proper alignment can improve memory access speed, particularly on architectures with stricter alignment requirements. Failure to align data structures correctly can lead to performance degradation, especially on systems with cache-sensitive applications. Case study: An image processing application experienced a significant speed boost after ensuring proper alignment of its pixel data structures. Another example: A high-performance computing application saw improved performance by aligning its numerical data structures properly, improving data locality. Careful planning and optimization of data structures can yield improvements.
Efficient use of memory pools can also reduce allocation overhead, particularly in scenarios involving frequent allocations and deallocations of small objects. Memory pools provide a pre-allocated block of memory from which objects are allocated, eliminating the need for repeated calls to the system allocator. Case study: A networking application using a memory pool for managing network packets significantly reduced allocation overhead and improved throughput. Another example: A game engine utilized a memory pool to allocate and deallocate game objects efficiently, preventing performance bottlenecks during gameplay. The use of memory pools can bring significant optimization in resource-intensive applications.
Concurrency and Shared Memory: Safe and Efficient Approaches
Rust's concurrency model, built on ownership and borrowing, prevents many data races and memory corruption issues. However, mastering advanced concurrency techniques is essential for building highly efficient and scalable applications. Case study: A web server utilizing channels and message passing for inter-thread communication ensured safe and efficient handling of concurrent requests. Another example: A distributed computing application employing futures and async/await for concurrent tasks improved scalability and resource utilization.
Understanding the trade-offs between different concurrency models, such as message passing and shared memory, is crucial for choosing the optimal approach for a given problem. Case study: A real-time system opted for message passing to ensure deterministic behavior and minimal latency. Another example: A database system leveraged shared memory for performance-critical operations to minimize inter-thread communication.
Efficient use of synchronization primitives, such as mutexes, semaphores, and channels, is paramount for avoiding deadlocks and race conditions. Case study: A multi-threaded application using mutexes effectively prevented data corruption and race conditions. Another example: A concurrent data structure employed semaphores for efficient resource control.
Exploring advanced concurrency patterns such as work-stealing queues, actor models, and channels can lead to significant performance improvements in specific use cases. Case study: A parallel processing application benefited from a work-stealing queue, dynamically distributing workloads across multiple threads. Another example: A distributed system leveraged an actor model to simplify concurrent programming and improve scalability.
Error Handling and Resource Management: Going Beyond `Result`
While `Result
Effective use of `panic!` for unrecoverable errors is an integral part of Rust's error handling strategy. Understanding when to use `panic!` versus returning an error is critical. Case study: A library utilized `panic!` for internal consistency violations, ensuring that inconsistencies would not propagate to the calling code. Another example: An application employed `panic!` for conditions indicating critical system failures. Proper use of `panic!` helps to maintain code integrity.
Implementing custom error types provides opportunities to improve the clarity and detail of error reporting. Case study: A library created custom error types for more informative error messages, helping developers quickly pinpoint problems. Another example: An application defined a hierarchy of custom error types to categorize various errors effectively. Custom errors provide more granular error handling.
Resource management techniques like RAII (Resource Acquisition Is Initialization) and smart pointers are fundamental to Rust's memory safety guarantees. However, the nuances of RAII and their implications for resource handling in complex scenarios often require in-depth understanding. Case study: A file-handling library relied on RAII to ensure proper file closure upon function completion, preventing resource leaks. Another example: A network application efficiently managed network sockets using RAII and smart pointers, preventing resource exhaustion. RAII improves safety and prevents memory leaks.
Profiling and Optimization: Advanced Techniques for Performance Tuning
Profiling is essential for identifying performance bottlenecks in Rust applications. Tools like `cargo flamegraph` and `perf` provide invaluable insights into code execution patterns. Case study: A developer used `cargo flamegraph` to identify a performance bottleneck in a sorting algorithm, leading to significant optimization. Another example: An application developer employed `perf` to profile system-level performance issues, revealing bottlenecks related to I/O operations. Profiling reveals areas for optimization.
Understanding compiler optimizations and their effects on code performance is crucial. Techniques like loop unrolling, inlining, and constant propagation can lead to noticeable speed improvements. Case study: A developer optimized a computationally intensive loop by applying loop unrolling, leading to a significant reduction in execution time. Another example: An application benefited from compiler inlining, reducing function call overhead and improving performance. Compiler optimizations can improve performance.
Efficient use of data structures and algorithms is fundamental to optimizing performance. Choosing the correct data structure for a particular task can have a significant impact on performance. Case study: A developer switched from a linked list to a vector, leading to substantial performance improvements in a data processing task. Another example: An application used a hash table instead of a linear search, reducing search time exponentially. Appropriate data structures impact performance.
Advanced optimization techniques like SIMD (Single Instruction, Multiple Data) vectorization can significantly enhance performance in computationally intensive tasks. Case study: A developer leveraged SIMD vectorization to accelerate image processing operations, leading to considerable speed improvements. Another example: A scientific computing application used SIMD instructions to speed up matrix multiplications dramatically. SIMD increases performance significantly.
In conclusion, mastering Rust's memory management goes beyond the basics. While the borrow checker provides a strong foundation, understanding advanced techniques like custom allocators, efficient concurrency strategies, and sophisticated error handling is crucial for building truly performant and robust applications. By embracing these advanced concepts, developers can create highly optimized Rust code that leverages the language's strengths to their fullest extent. The journey to becoming a proficient Rustacean involves continuous learning and exploration of these often-overlooked aspects of the language.