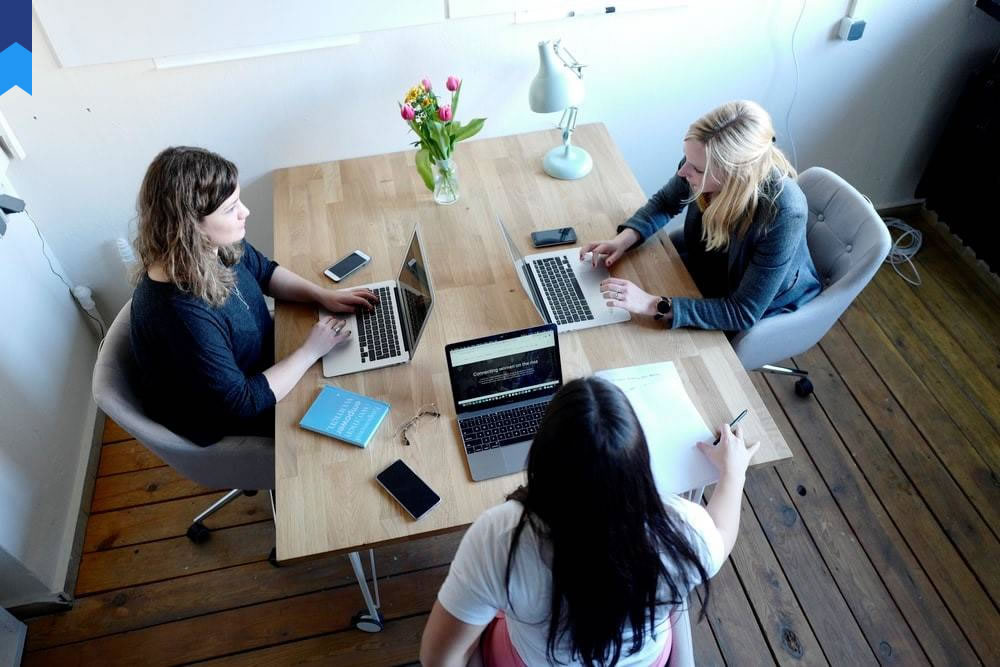
Unlocking ADO.NET: A Comprehensive Introduction to Data Access in the .NET Framework
Introduction
ADO.NET (ActiveX Data Objects for .NET) is a powerful and essential component of the .NET Framework, providing a robust set of features for data access in applications. Whether you're building web applications, desktop software, or services, understanding ADO.NET is fundamental to efficiently interact with databases and manage data. This comprehensive guide unlocks the intricacies of ADO.NET, offering a step-by-step introduction to its core components, best practices, and advanced features.
1. Understanding ADO.NET: Foundations and Core Concepts
Definition of ADO.NET:
ADO.NET is a set of .NET classes that facilitates data access, enabling applications to interact with diverse data sources, including relational databases, XML, and more. It provides a unified framework for managing and manipulating data, ensuring a seamless bridge between applications and databases.
Core Components:
Data Providers:
ADO.NET includes data providers specific to various databases, such as SQL Server, Oracle, MySQL, and more. These providers act as bridges between the application and the database.
DataSet:
ADO.NET's in-memory cache of data retrieved from a data source. The DataSet enables disconnected data access, allowing manipulation of data without a continuous connection to the database.
DataReader:
A forward-only, read-only cursor for efficiently retrieving data from a data source. DataReaders are suitable for scenarios where read-only, sequential access to data is sufficient.
DataAdapter:
Serves as a bridge between a DataSet and a data source, facilitating the two-way transfer of data. It consists of the DataCommand, which executes SQL commands, and the DataReader, which reads the results.
2. Establishing Database Connections with ADO.NET
Connection String Configuration:
Connection strings are crucial in establishing connections to databases. They contain information such as the database server's address, authentication details, and other parameters. Understanding connection string formats is essential for configuring connections.
Connecting to a Database:
```csharp
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "Data Source=ServerAddress;Initial Catalog=DatabaseName;User ID=Username;Password=Password";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// Perform database operations
}
}
}
```
3. Executing SQL Commands with ADO.NET
DataCommand and SQL Queries:
DataCommands execute SQL queries and commands. They play a central role in data access by facilitating interactions between the application and the database.
Executing a Query:
```csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string sqlQuery = "SELECT * FROM TableName";
using (SqlCommand command = new SqlCommand(sqlQuery, connection))
{
using (SqlDataReader reader = command.ExecuteReader())
{
// Process the results
}
}
}
```
4. Retrieving and Manipulating Data with DataReaders
Data Retrieval with DataReader:
DataReaders provide a lightweight, forward-only mechanism for retrieving data. They are efficient for scenarios where read-only access to data is sufficient.
Example of Data Retrieval:
```csharp
using (SqlDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
// Access data using reader["ColumnName"]
}
}
```
Disconnected Data Access with DataSets:
DataSets allow for disconnected data access, enabling manipulation of data without a continuous connection to the database. They consist of DataTables, each representing a table of data.
```csharp
using (SqlDataAdapter adapter = new SqlDataAdapter(sqlQuery, connection))
{
DataSet dataSet = new DataSet();
adapter.Fill(dataSet, "TableName");
// Access data using dataSet.Tables["TableName"]
}
```
5. Parameterized Queries for Security and Efficiency
Parameterized Queries:
Using parameterized queries is crucial for both security and performance. They help prevent SQL injection attacks and enhance query plan reuse.
Example of Parameterized Query:
```csharp
string sqlQuery = "SELECT * FROM TableName WHERE ColumnName = @ParamValue";
using (SqlCommand command = new SqlCommand(sqlQuery, connection))
{
command.Parameters.AddWithValue("@ParamValue", paramValue);
using (SqlDataReader reader = command.ExecuteReader())
{
// Process the results
}
}
```
6. Transactions and Error Handling in ADO.NET
Transactions for Data Integrity:
Transactions ensure data integrity by grouping multiple database operations into a single, atomic unit. In ADO.NET, transactions are managed using the `SqlTransaction` class.
Example of Transactions:
```csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
using (SqlTransaction transaction = connection.BeginTransaction())
{
try
{
// Perform database operations within the transaction
transaction.Commit();
}
catch (Exception ex)
{
transaction.Rollback();
// Handle the exception
}
}
}
```
Error Handling:
ADO.NET provides robust error handling mechanisms. By utilizing try-catch blocks, developers can gracefully handle exceptions that may occur during data access operations.
```csharp
try
{
// ADO.NET code
}
catch (SqlException ex)
{
// Handle SQL-specific exceptions
}
catch (Exception ex)
{
// Handle other exceptions
}
```
7. Asynchronous Data Access in ADO.NET
Asynchronous Programming with ADO.NET:
Asynchronous data access in ADO.NET improves application responsiveness by allowing tasks such as database queries to
run asynchronously without blocking the main thread.
Example of Asynchronous Query:
```csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
await connection.OpenAsync();
string sqlQuery = "SELECT * FROM TableName";
using (SqlCommand command = new SqlCommand(sqlQuery, connection))
{
using (SqlDataReader reader = await command.ExecuteReaderAsync())
{
// Process the results
}
}
}
```
8. Advanced ADO.NET: Stored Procedures and Views
Using Stored Procedures:
Stored procedures offer a way to encapsulate and execute pre-defined SQL queries on the database server. ADO.NET supports the execution of stored procedures.
```csharp
using (SqlCommand command = new SqlCommand("StoredProcedureName", connection))
{
command.CommandType = CommandType.StoredProcedure;
// Set parameters if needed
using (SqlDataReader reader = command.ExecuteReader())
{
// Process the results
}
}
```
Querying Database Views:
ADO.NET seamlessly handles queries involving database views. Views, which are virtual tables based on the result of a SELECT query, can be queried like regular tables.
```csharp
string sqlQuery = "SELECT * FROM DatabaseView";
using (SqlCommand command = new SqlCommand(sqlQuery, connection))
{
using (SqlDataReader reader = command.ExecuteReader())
{
// Process the results
}
}
```
9. Optimizing ADO.NET: Connection Pooling and Performance
Connection Pooling:
Connection pooling enhances performance by reusing existing database connections, reducing the overhead of creating and closing connections for each operation.
Optimizing Performance:
- Use connection pooling to minimize the overhead of opening and closing connections.
- Employ parameterized queries to enhance security and performance.
- Leverage asynchronous programming for improved responsiveness.
- Consider caching strategies for frequently accessed data.
Conclusion:
Mastering Data Access with ADO.NET
ADO.NET stands as a cornerstone for effective data access in .NET applications. From establishing connections and executing queries to handling transactions and optimizing performance, this guide provides a comprehensive introduction to mastering ADO.NET. By understanding its core components, adopting best practices, and exploring advanced features, developers can unlock the full potential of ADO.NET in building robust and efficient database-driven applications. As the .NET ecosystem evolves, ADO.NET continues to play a vital role in shaping the data access landscape for modern software development.
SIIT Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs