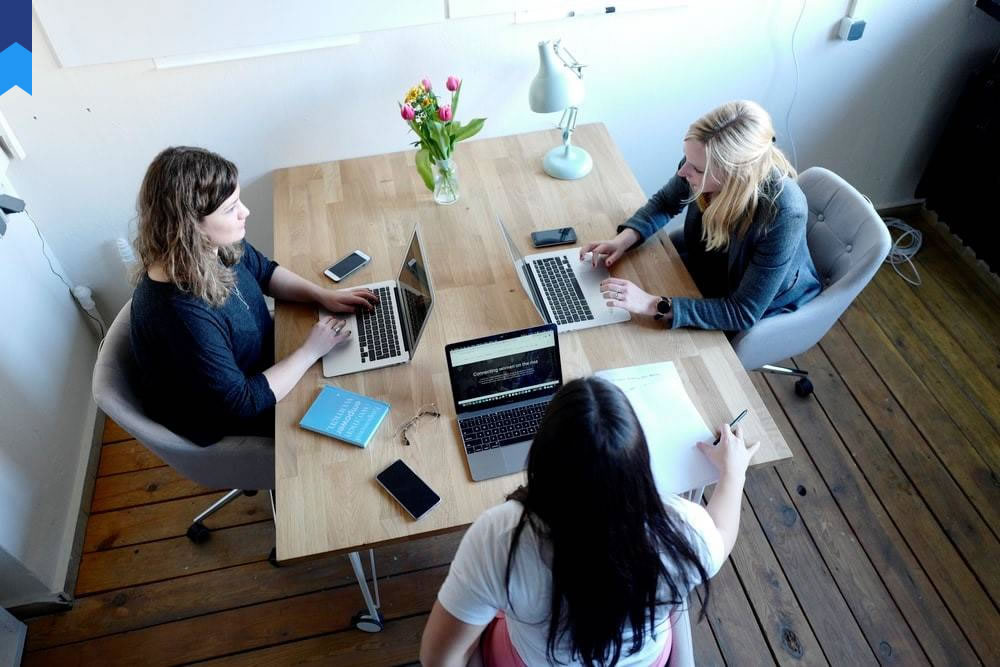
Mastering JavaScript Debugging: 10 Strategies For Efficient Problem-Solving
Coding errors – something that every developer hates.
And it is understandable!
Let's say you're using JavaScript to work on a website, and the form's "submit" button doesn't work.
The worst part? You are unable to precisely identify the error's main cause. That must be annoying, right?
How are you going to fix it? With debugging in JavaScript!
Whatever problems you're having, we can help you fix them. In this post, we'll talk about 10 useful tips for debugging in JavaScript.
What is JavaScript?
JavaScript is a computer language that lets you make websites and apps that people can interact with. One thing that makes it unique is that it works in your computer browser as well as on a server.
JavaScript, along with HTML and CSS, is what makes most of the internet work. Many websites (about 99%) actually use JavaScript.
For example, you can use JavaScript to build web and mobile apps, make websites more engaging, create web servers and server apps, develop games, and more.
The Angry Birds game that you play on your smartphone is created using JavaScript.
Now, you don't really need to master JavaScript to create interactive websites. With the help of AI website builder tools like Hocoos, you can easily create any type of website from scratch. All you need to do is answer 8 questions, and your site will be ready. What's best is that you don't have to worry about coding errors or debugging in JavaScript.
What is Debugging?
Debugging is a process used in computer programming and engineering to fix problems. It involves key steps like,
- Finding the issue
- Figuring out what's causing it
- Either fixing it or finding a way to work with it
- And testing everything to make sure it's working correctly
A study found that workers spend about 25% to 50% of their time fixing bugs.
Debugging in software development starts when a programmer finds a mistake in the code and can make the problem happen again.
Debugging is an important part of checking software and of making software in general.
What is Debugging in JavaScript?
If you're writing code in JavaScript and something goes wrong, you need to follow the debugging steps to fix it.
Finding and solving bugs in your JavaScript code is what debugging means in JavaScript. It is used to make sure that your web apps work perfectly.
Common JavaScript Bugs and Errors
There are a lot of bugs in JavaScript that app and website developers may run into.
Syntax Errors |
Mistakes in the code, such as missing punctuation or spelling errors. |
Variable Scope Issues |
Incorrect usage of variables, leading to unexpected behavior. |
Type Errors |
Operations performed on incompatible data types, like using a method on a non-object. |
Logical Errors |
Code runs without errors but doesn't produce the expected output due to flawed logic. |
Event Handling Problems |
Issues with how events like clicks or form submissions are handled, causing unexpected behavior. |
Asynchronous Code Bugs |
Problems with code execution order due to asynchronous operations, resulting in timing issues. |
DOM Manipulation Errors |
Errors caused by incorrect changes to the web page's structure. |
Browser Compatibility |
Differences in JavaScript behavior across web browsers, requiring specific handling for each. |
10 Powerful Strategies for Debugging in JavaScript
The debugging process may seem easy, but it is actually very time-consuming and complicated. But here are the 10 key strategies and tips you can use for successful debugging in JavaScript.
1. Understand the error message
Error messages are very helpful for fixing issues in your code. They tell you exactly what went wrong and where it happened. When you see an error, take the time to read it carefully and figure out what it is saying.
Let's see an example of an error message in JavaScript.
TypeError: Cannot read property 'toUpperCase' of undefined
at greet (script.js:3)
at script.js:7
This error (TypeError: Cannot read property 'toUpperCase' of undefined) means that the greet function is trying to use the toUpperCase method on something that doesn't exist (it's undefined).
- at greet (script.js:3): This shows that the error happened inside the greet function on line 3 of script.js, where toUpperCase was used on an undefined value.
- at script.js:7: This indicates that the greet function was called from line 7 of script.js, which caused the error.
To fix this, we should ensure that the value used with toUpperCase inside the greet function is not undefined before trying to use this method.
2. Employ basic code
Try simplifying your code to make it a bit clearer if you are having problems identifying the error. Concentrate on the region where the issue is occurring and eliminate any unneeded or challenging components. This might facilitate the problem's identification and provide a speedier fix.
function addNumbers(a, b) {
let sum = a + b;
return sum;
}
let result = addNumbers(3, 5);
console.log(result);
In this instance, the addNumbers method adds the two integers. Code simplification like this may make errors easier to notice and rectify more quickly.
3. Use a debugger
Debuggers help programmers detect and correct errors in their code. The built-in debugger in JavaScript allows you to walk through your code and watch how the numbers change at each step.
Additionally, debugging tools for JavaScript are available in most modern web browsers, allowing you to instantly repair flaws in your code.
function calculateSquare(num) {
let square = num * num;
debugger;
return square;
}
let result = calculateSquare(5);
console.log(result);
By stopping the execution of the code at a certain line (debugger), we are using the debugger to investigate the value of the square variable in this instance. This makes finding and fixing any coding problems easier.
4. Console logging
One helpful way to find JavaScript bugs is to include console.log lines. At certain places in your code, you may examine the values and variables that your application is using by putting console.log. Now it's easy to see possible trouble spots.
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet('Alice');
The welcome function sends a greeting using the console and the supplied name argument. By utilizing console.log, we can ensure that the function obtains and publishes accurate data.
5. Setting breakpoints
Breakpoints are a quicker and more effective way to debug JavaScript than using console.log() commands. Breakpoints make it easy to examine the values of variables while the program is executing by enabling the user to halt the code at certain points.
function calculateSum(a, b) {
let result = a + b;
debugger;
// Set a breakpoint here
return result;
}
let x = calculateSum(2, 3);
console.log(x);
To make the calculateSum function fail, we've included a debugger; statement. The code will halt at this breakpoint during execution, allowing you to examine the values of the a, b, and result variables using the Developer Tools.
6. Source maps
Source maps are helpful files because they show the way back to the original source code of translated JavaScript code. They make it easier to debug by letting you set breakpoints and run code line by line.
Source maps improve error reporting by providing stack traces, error messages that connect to the underlying source files, and other such information. Finding and fixing bugs in production code becomes much easier with this.
Watch expressions
When debugging in JavaScript, watch expressions are helpful for keeping track of variable values or expressions as your code runs.
Therefore, what is the purpose of using watch expressions?
- Monitor the values of the variables as you fix them.
- Pay attention to and assess expressions.
- To check for specific occurrences, use conditional watches.
- Maintain the dashboard's integrity while keeping tabs on critical data points as they change in real time.
You may focus on and keep an eye on critical data in your code with the help of watch expressions. Because of this, even the most intricate patterns are easier to understand and fix.
7. Exception handling
One reliable method for finding JavaScript vulnerabilities is to use exception handling. If your code encounters issues while running, this method will help you fix them.
See this code snippet for an illustration of the try...catch to catch exceptions
try {
let result = 10 / 0;
console.log(result);
} catch (error) {
console.error("An error occurred:", error.message);
}
Here, we see an example of how to use try...catch to avoid application crashes and manage errors in a controlled way.
8. Code linting
Use programs like JSHint or ESLint to find and fix mistakes and guarantee correctness and regulatory compliance. By ensuring consistency, liners preserve code quality and identify frequent problems.
They help to produce code that is more error-free and succinct by pointing out problems like missing semicolons, unneeded variables, and bad grammar.
9. Unit testing
Using frameworks like Jasmine, Jest or Mocha helps simplify the process of creating tests to verify the functionality of your code. Unit tests examine particular code components (units) to confirm their intended functioning.
Preemptively writing tests enables the swift detection of problems, enhances the code's quality, and ensures the reliability of programs.
Final Thoughts
Before you can work on web development problems, you need to know how to debug JavaScript. You might be able to quickly find and fix problems with unit tests, troubleshooting tools, and interface logs. Also, using the right coding techniques, like error handling and code linting, makes the code better.