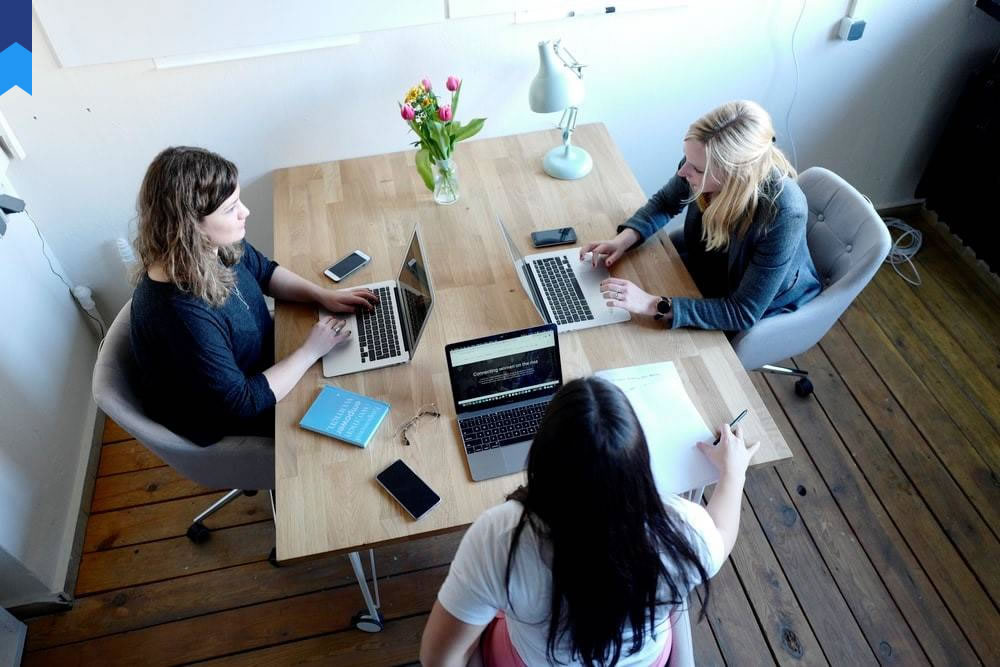
How Laravel Works: A Dummy’s Guide to Hidden Truths
Laravel is a powerful and flexible PHP framework designed to make web development easier and more efficient. It's built on the MVC (Model-View-Controller) architecture, which separates the application logic from the presentation layer, making the code more organized and maintainable. Laravel is known for its elegant syntax, comprehensive documentation, and robust feature set, making it an excellent choice for both beginners and experienced developers. If you're new to Laravel, this guide on laravel for dummies is for you. Whether you're an individual developer or part of a Laravel web development company, understanding the fundamentals of Laravel will set you on the path to building powerful web applications.
What is Laravel?
Laravel is an open-source PHP framework that provides tools and resources for building modern web applications. Created by Taylor Otwell, Laravel aims to take the pain out of development by easing common tasks like routing, authentication, sessions, and caching. For those working with a laravel web development company, these features streamline the development process, allowing for more efficient and maintainable code.
Key Features of Laravel
- Elegant Syntax: Laravel's syntax is expressive and elegant, making coding enjoyable and straightforward.
- MVC Architecture: The MVC pattern helps organize your code and separates business logic from the presentation layer.
- Eloquent ORM: Laravel's Object-Relational Mapping (ORM) makes database interactions easy and intuitive.
- Blade Templating: A powerful templating engine that simplifies the creation of dynamic views.
- Comprehensive Documentation: Laravel's documentation is thorough and beginner-friendly.
Setting Up Laravel
Before diving into coding, you need to set up your development environment. This section will guide you through the requirements and installation process.
System Requirements
To run Laravel, your server must meet the following requirements:
- PHP >= 7.3
- OpenSSL PHP Extension
- PDO PHP Extension
- Mbstring PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
- Ctype PHP Extension
- JSON PHP Extension
Installation Process
- Install Composer: Laravel uses Composer, a dependency manager for PHP. You can download it from getcomposer.org.
- Create a Laravel Project: Run the command composer create-project --prefer-dist laravel/laravel project-name to create a new Laravel project.
- Set Up a Database: Configure your database settings in the .env file located in the root directory of your project.
Configuration Basics
Laravel's configuration files are located in the config directory. You can configure various aspects of your application, including database connections, mail settings, and third-party services.
Understanding MVC Architecture
Laravel follows the MVC (Model-View-Controller) architectural pattern, which separates an application into three main logical components: the model, the view, and the controller.
Model-View-Controller (MVC) Explained
- Model: Represents the data layer and is responsible for managing the business logic and database interactions.
- View: Represents the presentation layer and is responsible for displaying data to the user.
- Controller: Acts as an intermediary between the model and the view. It handles user input and updates the model and view accordingly.
Benefits of Using MVC
- Separation of Concerns: MVC separates the application logic from the user interface, making the code more organized and maintainable.
- Reusability: Components can be reused across different parts of the application.
- Scalability: The application can be scaled more efficiently by separating concerns.
Routing in Laravel
Routing is a core aspect of any web application. Laravel's routing system is simple yet powerful, allowing you to define your application's routes in a straightforward manner.
Basic Routing
Routes are defined in the routes/web.php file. A basic route can be defined as follows:
Route::get('/', function () {
return view('welcome');
});
Route Parameters
You can define dynamic routes that accept parameters:
Route::get('user/{id}', function ($id) {
return 'User '.$id;
});
Named Routes
Named routes allow you to generate URLs or redirects for specific routes. You can name a route using the name method:
Route::get('profile', 'UserController@showProfile')->name('profile');
Blade Templating Engine
Blade is Laravel's powerful templating engine, providing a clean and efficient way to manage your application's views.
Introduction to Blade
Blade allows you to use plain PHP code in your templates and provides a number of shortcuts for common tasks. Blade files use the .blade.php extension and are stored in the resources/views directory.
Blade Syntax and Directives
Blade provides various directives to simplify view creation:
- @if, @else, @elseif, and @endif
- @for, @foreach, @forelse, and @endfor
- @include
Layouts and Sections
Blade's layout system allows you to define a master layout and extend it in your views. This promotes code reusability and consistency.
<!-- resources/views/layouts/app.blade.php -->
<html>
<head>
<title>App Name - @yield('title')</title>
</head>
<body>
@section('sidebar')
This is the master sidebar.
@show
<div class="container">
@yield('content')
</div>
</body>
</html>
<!-- resources/views/child.blade.php -->
@extends('layouts.app')
@section('title', 'Page Title')
@section('sidebar')
@parent
<p>This is appended to the master sidebar.</p>
@endsection
@section('content')
<p>This is my body content.</p>
@endsection
Database Management with Eloquent ORM
Eloquent ORM provides a simple and elegant way to interact with your database.
Introduction to Eloquent
Eloquent allows you to interact with your database using models. Each model represents a table in your database.
Migrations and Schema Building
Migrations provide a version control system for your database, allowing you to modify and share your database schema. You can create a migration using the make:migration Artisan command.
Basic CRUD Operations
Eloquent makes CRUD operations straightforward:
- Create: $user = User::create(['name' => 'John']);
- Read: $users = User::all();
- Update: $user->update(['name' => 'Jane']);
- Delete: $user->delete();
Middleware and Security
Middleware provides a convenient mechanism for filtering HTTP requests entering your application.
Understanding Middleware
Middleware acts as a bridge between a request and a response. Laravel includes several middleware, such as authentication and CSRF protection.
Implementing Middleware
You can create custom middleware using the make:middleware Artisan command. Middleware can be applied globally, to specific routes, or to route groups.
Security Best Practices
- Validation: Always validate user input.
- CSRF Protection: Laravel includes CSRF protection by default.
- Encryption: Use Laravel's built-in encryption services to secure sensitive data.
Handling Requests and Responses
Understanding the request lifecycle and handling responses efficiently is crucial for building robust applications.
Request Lifecycle
Laravel's request lifecycle begins when a request enters your application and ends when a response is sent back to the client.
Handling Form Requests
Laravel provides a convenient way to handle form validation and authorization using form request classes.
Generating Responses
You can generate different types of responses, including views, JSON, and redirects.
Laravel Artisan Command Line
Artisan is Laravel's powerful command-line interface, providing various commands to assist you during development.
Common Artisan Commands
- php artisan serve: Start the development server.
- php artisan migrate: Run database migrations.
- php artisan make:model ModelName: Create a new model.
Creating Custom Commands
You can create custom Artisan commands using the make:command Artisan command.
Testing in Laravel
Laravel provides a robust testing environment, allowing you to test your application thoroughly.
Introduction to Testing
Laravel supports both unit and feature testing out of the box. Tests are stored in the tests directory.
Unit Testing
Unit tests focus on individual units of code. You can create a unit test using the make:test Artisan command.
Feature Testing
Feature tests focus on larger portions of your application, such as HTTP requests and responses. You can create a feature test using the make:test Artisan command.
Deploying a Laravel Application
Deploying a Laravel application involves preparing your code and environment for production.
Preparing for Deployment
- Environment Configuration: Ensure your .env file is configured correctly.
- Caching: Cache your configuration and routes for improved performance.
Deployment Options
- Shared Hosting: Suitable for small applications.
- Cloud Hosting: Services like AWS, DigitalOcean, and Linode offer scalable hosting solutions.
Post-Deployment Checklist
- Database Migrations: Run pending migrations.
- Caching: Clear and regenerate caches.
- Backups: Set up regular backups.
Conclusion
In this guide, we've covered the essentials of Laravel, from setup to deployment. Whether you're just starting with Laravel for dummies or looking to outsource laravel development to a Laravel web development company, understanding these hidden truths will help you build robust and efficient web applications. By mastering these core concepts, you'll be well-equipped to leverage the power of Laravel in your projects.
Related Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs