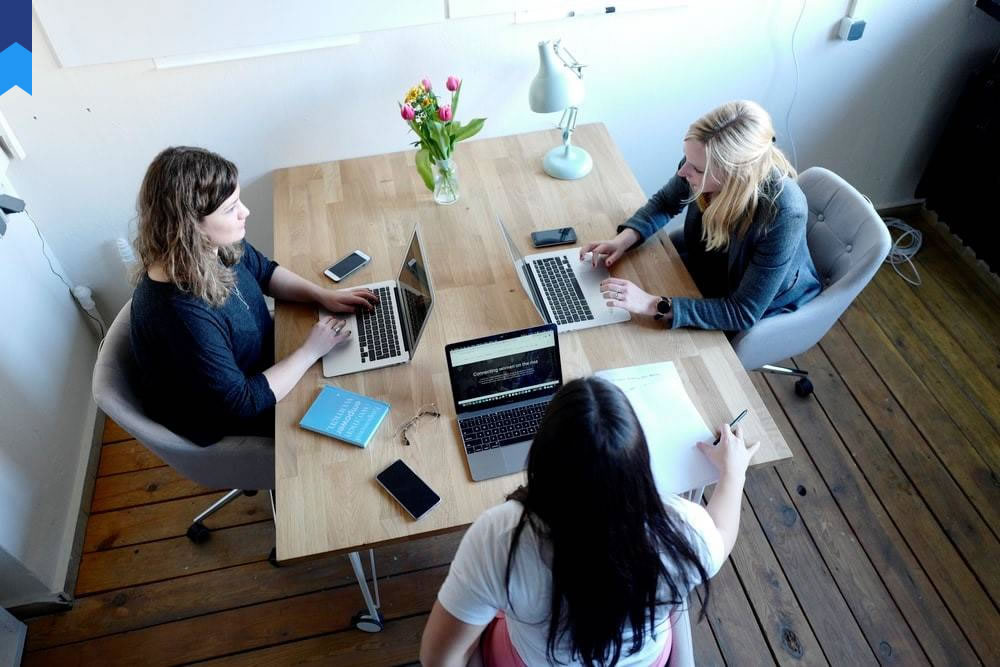
How To Implement Bing AI For Emergency response system
Implementing Bing AI for an emergency response system can significantly enhance the efficiency and effectiveness of disaster management, public safety, and real-time crisis response. Leveraging Bing AI’s capabilities in machine learning, natural language processing (NLP), and real-time data analytics, emergency response systems can automate information gathering, predict risks, optimize resource allocation, and improve communication during emergencies.
This guide will explore how to implement Bing AI in an emergency response system, covering key features, technologies, and steps required for building an AI-powered solution.
Overview of Emergency Response Systems with Bing AI
Emergency response systems deal with critical situations such as natural disasters, accidents, or public safety threats.
Bing AI can help:
1. Analyze real-time data from multiple sources (social media, news, sensors).
2. Provide automated alerts to emergency services and the public.
3. Predict risks and potential impacts of disasters.
4. Optimize response time and resource allocation (e.g., first responders, medical supplies).
5. Enhance communication and coordination among emergency teams.
Key Components of a Bing AI-powered Emergency Response System
The following are key tools and technologies to build an emergency response system using Bing AI:
1. Bing Search API: To monitor and retrieve real-time data from news articles, social media, and web sources.
2. Azure Cognitive Services: For sentiment analysis, image recognition, and speech-to-text capabilities.
3. Azure Machine Learning: To predict disaster risks, model emergency scenarios, and optimize response strategies.
4. IoT Sensors and Networks: To gather environmental data (e.g., weather, traffic) and automate alerts.
5. Communication platforms: To send automated alerts via SMS, email, or mobile apps to citizens and responders.
Steps to Implement Bing AI for Emergency Response
Real-Time Data Collection and Monitoring
An emergency response system needs to collect and monitor real-time data from various sources, including public reports, weather sensors, traffic systems, and social media. Bing AI’s search capabilities allow for continuous data gathering from diverse platforms.
1. Using Bing Search API for Real-time Monitoring: Bing AI can gather information from news articles, web sources, and public social media posts during an emergency. This data helps responders stay informed about real-time conditions.
import requests
def gather_emergency_data(query):
# Example of using Bing Search API to retrieve real-time information
subscription_key = "your_bing_search_key"
url = f"https://api.bing.microsoft.com/v7.0/search?q={query}"
headers = {"Ocp-Apim-Subscription-Key": subscription_key}
response = requests.get(url, headers=headers)
return response.json()
# Example: Monitoring news for earthquake-related emergencies
emergency_data = gather_emergency_data("earthquake near city")
print(emergency_data)
This enables first responders to stay up-to-date with unfolding events and make informed decisions on-the-fly.
AI-driven Risk Prediction and Disaster Modeling
Bing AI can analyze historical and real-time data to predict the likelihood of certain disasters (e.g., floods, wildfires) and model potential impacts. This helps emergency managers plan and allocate resources more efficiently.
1. Using Machine Learning for Disaster Risk Prediction: Train machine learning models on historical data (e.g., past earthquakes, fires) to predict the probability and severity of future emergencies.
from azure.ai.ml import MLClient
from azure.ai.ml.models import AutoMLJob
def predict_disaster_risk(disaster_data):
# Example AI model for predicting risk of disasters like floods or fires
ml_client = MLClient(subscription_id="your_subscription_id")
job = AutoMLJob(task="forecasting", primary_metric="accuracy")
return job.run(disaster_data)
# Example: Predicting the risk of flooding based on weather patterns
flood_risk = predict_disaster_risk(weather_data)
print(flood_risk)
AI-driven risk predictions enable emergency managers to allocate resources where they’re needed most and prepare for potential emergencies before they escalate.
Automated Alerts and Response Coordination
In an emergency, timely alerts and communication are critical. Bing AI can automate alert systems based on pre-defined triggers, such as weather patterns, social media sentiment, or sensor data, and send notifications to emergency teams and the public.
1. Setting Up Automated Alerts: Use Azure Logic Apps to trigger automated alerts and notifications when specific emergency criteria are met.
from azure.identity import DefaultAzureCredential
from azure.logicapps import LogicAppsClient
def send_alert(alert_message):
# Example of sending automated alerts when disaster conditions are detected
logic_client = LogicAppsClient(credential=DefaultAzureCredential())
trigger = logic_client.workflow_triggers.create_or_update(
resource_group_name="emergency_response_group",
workflow_name="send_alerts_workflow",
trigger_name="alert_trigger",
body={"message": alert_message}
)
return trigger
# Example: Sending an earthquake alert
send_alert("Earthquake detected near city. Evacuate immediately.")
This ensures that emergency alerts are sent out rapidly to reduce response times and keep citizens informed.
Real-time Data Analysis for Resource Allocation
Bing AI can also analyze real-time data to determine which resources (medical personnel, fire trucks, evacuation routes) should be deployed to different areas based on the severity of the emergency.
1. AI-driven Resource Allocation: Use machine learning algorithms to analyze the severity of an emergency and optimize the deployment of resources.
def allocate_resources(emergency_data):
# AI-driven resource allocation logic
if emergency_data['severity'] == 'high':
return "Deploy additional medical teams and firefighters"
else:
return "Deploy minimal resources"
# Example: Allocating resources for a wildfire emergency
resource_allocation = allocate_resources({'severity': 'high'})
print(resource_allocation)
This ensures that resources are deployed where they’re most needed, reducing damage and saving lives.
Natural Language Processing (NLP) for Incident Reporting
During an emergency, it’s important to quickly process reports from the public, media, and emergency services. Bing AI’s NLP capabilities can analyze text reports, extract key information, and summarize incidents for faster response.
1. Processing Incident Reports: Use Azure Cognitive Services to process written or spoken reports from the public or first responders, automatically generating summaries and extracting critical information.
from azure.ai.textanalytics import TextAnalyticsClient
from azure.core.credentials import AzureKeyCredential
def process_incident_report(report_text):
# Example of using NLP to process emergency incident reports
client = TextAnalyticsClient(endpoint="your_endpoint", credential=AzureKeyCredential("your_key"))
response = client.extract_key_phrases([report_text])
return response[0].key_phrases
# Example: Processing a report from a first responder
incident_summary = process_incident_report("A fire has broken out in the north sector, evacuating residents.")
print(incident_summary)
This allows emergency managers to quickly assess situations and prioritize responses based on summarized reports.
Crowdsourced Emergency Reporting and Public Feedback
Bing AI can also help integrate crowdsourced data into emergency response systems, enabling the public to report emergencies and provide real-time updates through social media or mobile apps.
1. Crowdsourced Data Integration: Allow citizens to submit reports or provide feedback on conditions in their area, and use Bing AI to analyze and map this information.
def integrate_public_reports(report):
# Example of crowdsourcing emergency reports
return f"Report from {report['location']}: {report['description']}"
# Example: Integrating a public report of a roadblock during a flood
public_report = integrate_public_reports({"location": "Downtown", "description": "Road blocked due to flooding"})
print(public_report)
Crowdsourcing allows for faster identification of emergency zones and helps first responders understand the evolving conditions.
Testing and Optimizing the System
Testing and optimizing your emergency response system is crucial to ensure it operates effectively during real-world emergencies.
Steps include:
1. Simulating emergencies: Run simulations to test how well the system responds to different types of crises, such as natural disasters, accidents, or public safety threats.
2 Continuous learning: Refine AI models with new data to improve prediction accuracy and resource allocation over time.
3. User feedback: Collect feedback from first responders and the public to optimize the system’s alerts, reporting interfaces, and overall functionality.
Examples of AI-driven Emergency Response Systems
1. Natural Disaster Management: Bing AI can predict natural disasters like hurricanes or earthquakes, send out early warnings, and coordinate rescue efforts.
2. Traffic Incident Management: Real-time traffic and accident data can be used to redirect emergency vehicles, optimize evacuation routes, and prevent road congestion during emergencies.
3. Public Health Emergencies: During health crises like pandemics, AI can track infection rates, predict outbreaks, and recommend strategies for medical supply allocation and public safety.
Challenges and Ethical Considerations
When implementing an AI-powered emergency response system, there are several challenges and ethical considerations to keep in mind:
1. Data Privacy: Ensure that personal data from citizens and first responders is protected and only used for emergency purposes.
2. Accuracy and Reliability: AI models need to be accurate and reliable to avoid false alarms or missed emergencies.
3. Bias in AI Models: AI models should be trained on diverse datasets to prevent bias in predicting risks or allocating resources unfairly.
Conclusion
Implementing Bing AI in an emergency response system can dramatically enhance the speed, accuracy, and effectiveness of emergency management.
Related Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs