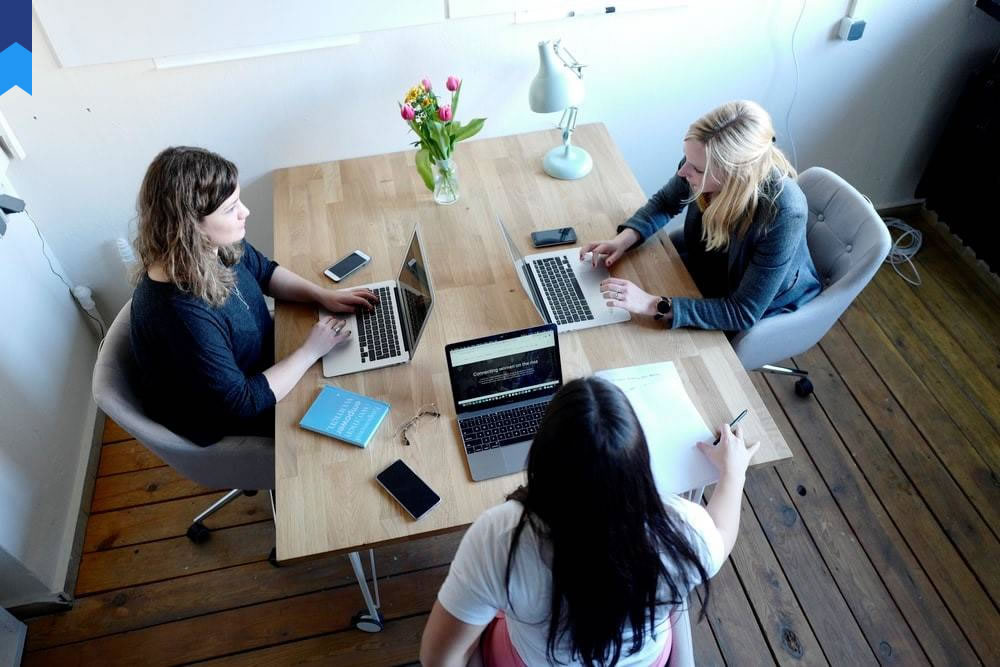
How To Create Bing AI-based Personal Finance Planners
Creating a Bing AI-based personal finance planner can revolutionize the way individuals manage their finances by offering tailored financial advice, real-time insights, and predictive analytics. This guide will walk you through the steps to develop a personal finance planner using Bing AI, focusing on features such as budget management, expense tracking, financial goal-setting, and investment planning.
Introduction to AI in Personal Finance
AI-powered personal finance tools leverage machine learning and natural language processing (NLP) to automate financial tasks, offer personalized advice, and analyze user data.
With Bing AI, you can build a financial planner that helps users:
1. Track income and expenses.
2. Set and achieve financial goals.
3. Create personalized budgets.
4. Provide investment suggestions.
5. Generate financial reports.
By using AI, the finance planner can learn from the user’s financial habits and provide smarter, more personalized advice over time.
Key Features of a Bing AI-based Personal Finance Planner
A comprehensive AI-based personal finance planner should include the following features:
1. Budget Tracking: Real-time tracking of income and expenses with intelligent categorization.
2. Expense Management: Analyzing spending patterns and offering suggestions for savings.
3. Goal Setting: Helping users set financial goals, such as saving for a home or paying off debt.
4. Predictive Analysis: Using historical data to predict future financial trends.
5. Investment Planning: Providing advice on savings, investments, and portfolio management.
6. Debt Management: Suggesting strategies to manage and pay off debts efficiently.
Steps to Create a Bing AI-based Personal Finance Planner
Define Financial Categories and Data Structure
Start by defining the financial categories that the planner will manage.
These may include:
1. Income: Salary, freelance payments, investments.
2 Expenses: Groceries, rent, utilities, entertainment, loans.
3. Savings and Investments: Emergency fund, retirement account, stocks, bonds.
Next, create a data structure that will capture this information in an organized way, using a database or structured format.
For example:
import pandas as pd
# Define financial categories and data structure
data = {
'category': ['Income', 'Rent', 'Groceries', 'Utilities', 'Investments'],
'amount': [5000, 1200, 400, 200, 1000],
'type': ['income', 'expense', 'expense', 'expense', 'investment']
}
# Create a dataframe
df = pd.DataFrame(data)
print(df)
This data structure will serve as the foundation for AI-based analysis and recommendations.
Expense and Budget Tracking with AI
A key feature of a personal finance planner is tracking expenses and comparing them to a budget. Bing AI can categorize expenses automatically and provide insights into spending habits.
Example of categorizing expenses:
def categorize_expense(description):
if 'rent' in description.lower():
return 'Rent'
elif 'grocery' in description.lower():
return 'Groceries'
elif 'utility' in description.lower():
return 'Utilities'
else:
return 'Miscellaneous'
# Example expense description
expense_description = "Paid for groceries at the store"
category = categorize_expense(expense_description)
print(f"Expense Category: {category}")
The AI model can learn from the user’s spending patterns and adjust the categorization for more accurate tracking over time.
Goal Setting and Financial Planning
An AI-powered finance planner can help users define financial goals, such as saving for a vacation or paying off debt, and track progress. Bing AI can use machine learning models to estimate how long it will take to reach these goals based on income and spending patterns.
Example of setting a financial goal:
def calculate_savings_goal(current_savings, monthly_contribution, target_amount):
months_needed = (target_amount - current_savings) / monthly_contribution
return round(months_needed, 2)
# Define a goal
current_savings = 2000
monthly_contribution = 300
target_amount = 5000
months_needed = calculate_savings_goal(current_savings, monthly_contribution, target_amount)
print(f"Months needed to reach the goal: {months_needed}")
Bing AI can further suggest ways to optimize savings by identifying areas where users can cut down on unnecessary expenses.
Predictive Analysis for Financial Trends
Bing AI can analyze past financial data and make predictions about future expenses and savings. This allows users to see trends such as when they might run into financial trouble or when they will meet a savings goal.
Example of using past data to predict future trends:
import numpy as np
# Example past expense data
past_expenses = np.array([500, 450, 600, 400, 550])
# Predict next month's expense using simple moving average
next_month_expense = np.mean(past_expenses[-3:])
print(f"Predicted expense for next month: {next_month_expense}")
More advanced models could use time-series analysis or machine learning to improve prediction accuracy.
Investment and Portfolio Management
Bing AI can offer tailored investment advice based on user financial goals, risk tolerance, and current market trends. It can also provide portfolio management services by suggesting the right mix of stocks, bonds, or other assets.
For example, a simple AI-powered suggestion for investment diversification might be:
def recommend_investment_portfolio(risk_tolerance):
if risk_tolerance == 'high':
return {'Stocks': 70, 'Bonds': 20, 'Real Estate': 10}
elif risk_tolerance == 'medium':
return {'Stocks': 50, 'Bonds': 30, 'Real Estate': 20}
else:
return {'Stocks': 30, 'Bonds': 50, 'Real Estate': 20}
# Example risk tolerance
portfolio_recommendation = recommend_investment_portfolio('medium')
print(f"Recommended portfolio: {portfolio_recommendation}")
Over time, Bing AI can refine these recommendations based on the user’s portfolio performance and market changes.
Debt Management
Debt management is crucial for personal finance. Bing AI can help users prioritize debt repayment strategies, such as the avalanche or snowball methods, depending on the type of debt and interest rates.
For example, recommending a debt repayment plan:
def recommend_debt_repayment(debts):
# Sort debts by interest rate for avalanche method
return sorted(debts, key=lambda x: x['interest_rate'], reverse=True)
# Example debts
debts = [
{'name': 'Credit Card', 'balance': 2000, 'interest_rate': 0.18},
{'name': 'Student Loan', 'balance': 15000, 'interest_rate': 0.04},
{'name': 'Car Loan', 'balance': 8000, 'interest_rate': 0.07}
]
repayment_plan = recommend_debt_repayment(debts)
print("Debt repayment order:", repayment_plan)
Bing AI can then track the user’s debt payments and suggest adjustments to stay on course.
Building the User Interface
Once the backend for Bing AI-powered financial planning is built, you’ll need to create a user-friendly interface that allows users to interact with the AI.
This can be done through:
1. Web applications: Using HTML/CSS and JavaScript for front-end design.
2. Mobile applications: Offering a responsive design for on-the-go financial tracking.
3. Chatbots: Users can interact with AI through natural language, asking questions like, “How much can I save this month?” or “What’s my current budget status?”
Example of chatbot interaction:
def chatbot_response(user_input):
if 'budget' in user_input.lower():
return "Your current budget balance is $500."
elif 'goal' in user_input.lower():
return "You are on track to save $3000 in 6 months."
else:
return "I'm here to help you with your finances."
# Example user input
user_input = "How's my budget?"
response = chatbot_response(user_input)
print(response)
Ensuring Data Security and Privacy
Since personal finance data is sensitive, ensure that your AI-powered finance planner follows strict security protocols:
1. Data encryption: Protect user data with encryption both at rest and in transit.
2. Compliance with regulations: Ensure the platform complies with financial regulations, such as GDPR or the U.S. Privacy Act.
3. Authentication: Implement secure authentication, such as two-factor authentication (2FA), to protect user accounts.
Conclusion
Creating a Bing AI-based personal finance planner offers users a powerful tool for managing their finances, setting goals, and making informed decisions about saving, spending, and investing. By leveraging AI’s capabilities in data analysis, budget tracking, predictive modeling, and investment management, you can deliver personalized and efficient financial planning services. As you build your solution, focus on data security, user experience, and continually improving AI models for better financial insights.
Related Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs